JavaScript: Get all prime numbers from 0 to a specified number
JavaScript Math: Exercise-43 with Solution
Write a JavaScript function to get all prime numbers from 0 to a specified number
Test Data:
console.log(primeFactorsTo(5));
[2, 3, 5]
console.log(primeFactorsTo(15));
[2, 3, 5, 7, 11, 13]
Visual Presentation:
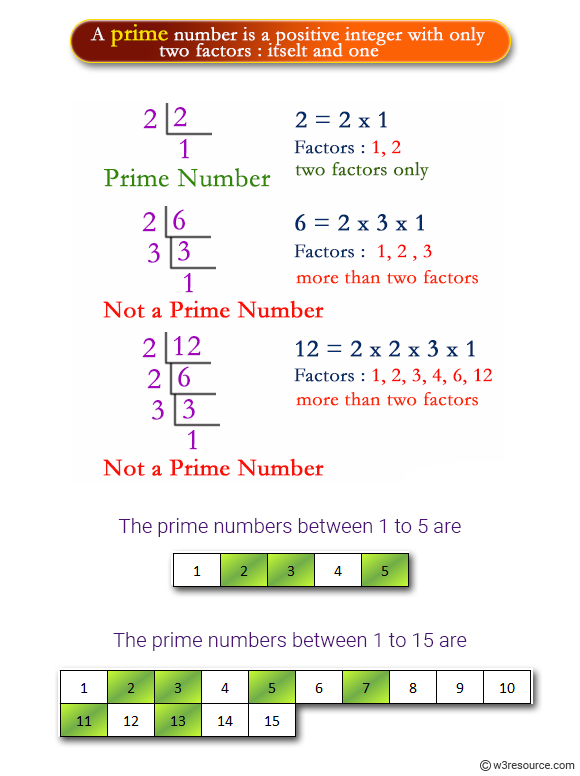
Sample Solution-1:
JavaScript Code:
// Define a function named primeFactorsTo that generates an array of prime numbers up to a given maximum value.
function primeFactorsTo(max)
{
// Initialize an array to store whether each number is prime.
var store = [],
i, j,
primes = [];
// Iterate through numbers from 2 to the maximum value.
for (i = 2; i <= max; ++i)
{
// If the current number is not marked as composite (not prime), add it to the primes array.
if (!store[i])
{
primes.push(i);
// Mark all multiples of the current prime as composite.
for (j = i << 1; j <= max; j += i)
{
store[j] = true;
}
}
}
// Return the array of prime numbers.
return primes;
}
// Output the result of finding prime numbers up to 5 to the console.
console.log(primeFactorsTo(5));
// Output the result of finding prime numbers up to 15 to the console.
console.log(primeFactorsTo(15));
Output:
[2,3,5] [2,3,5,7,11,13].
Flowchart:
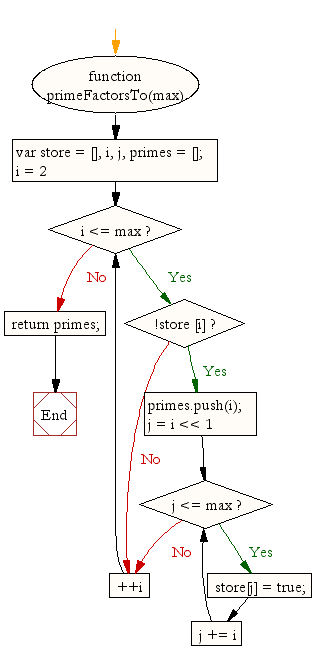
Sample Solution-2:
Generates primes up to a given number, using the Sieve of Eratosthenes.
- Generate an array from 2 to the given number.
- Use Array.prototype.filter() to filter out the values divisible by any number from 2 to the square root of the provided number.
JavaScript Code:
// Define a function named primes that generates an array of prime numbers up to a given number.
// Source: https://bit.ly/3hEZdCl
const primes = num => {
// Create an array of numbers from 2 to 'num'.
let arr = Array.from({ length: num - 1 }).map((x, i) => i + 2),
// Calculate the square root of 'num'.
sqroot = Math.floor(Math.sqrt(num)),
// Create an array of numbers from 2 to the square root of 'num'.
numsTillSqroot = Array.from({ length: sqroot - 1 }).map((x, i) => i + 2);
// Iterate through each number from 2 to the square root of 'num'.
numsTillSqroot.forEach(x => (arr = arr.filter(y => y % x !== 0 || y === x)));
// Return the array of prime numbers.
return arr;
};
// Output the result of generating prime numbers up to 5 to the console.
console.log(primes(5));
// Output the result of generating prime numbers up to 10 to the console.
console.log(primes(10));
// Output the result of generating prime numbers up to 15 to the console.
console.log(primes(15));
Output:
[2,3,5] [2,3,5,7] [2,3,5,7,11,13]
Flowchart:
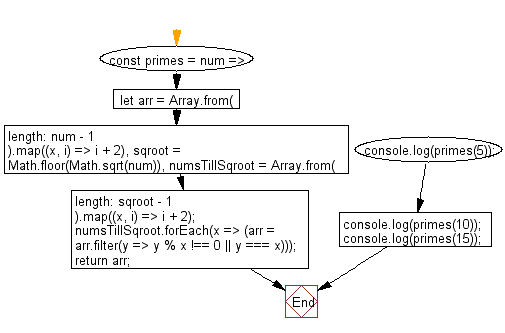
Live Demo:
See the Pen javascript-math-exercise-43 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to calculate the combination of n and r.
Next: Write a JavaScript function to show the first twenty Hamming numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics