JavaScript: Swap two variables
JavaScript Math: Exercise-55 with Solution
Swap Variables
Write a JavaScript program to swap variables from one to another.
Swapping two variables refers to mutually exchanging the values of the variables. Generally, this is done with the data in memory.
The simplest method to swap two variables is to use a third temporary variable :
define swap(x, y)
temp := x
x := y
y := temp
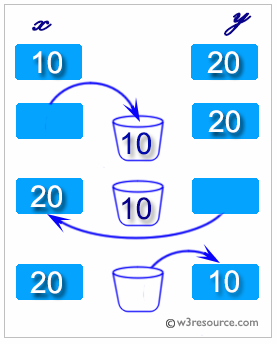
Sample Solution:
JavaScript Code:
// Declare and initialize two variables, x and y, with the values 20 and 40, respectively.
let x = 20;
let y = 40;
// Output the values of x and y.
console.log("x="+x+" y="+y)
// Declare a temporary variable and swap the values of x and y.
let temp;
temp = x;
x = y;
y = temp;
// Output the values of x and y after swapping.
console.log("After swapping:")
console.log("x="+x +" y="+y)
Output:
"x=" 20 "y=" 40 "After swapping:" "x=" 40 "y=" 20
Flowchart:
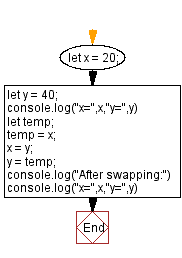
Live Demo:
See the Pen javascript-math-exercise-55 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that swaps two variables using array destructuring without a temporary variable.
- Write a JavaScript function that swaps two numeric variables using arithmetic operations only.
- Write a JavaScript function that swaps variables using bitwise XOR and verifies the swap with test outputs.
- Write a JavaScript function that swaps three variables cyclically without using any additional storage.
Improve this sample solution and post your code through Disqus.
Previous: Check if a given number is a power of 10.
Next: Volume of a Cuboid.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.