JavaScript: Volume of a Cone
JavaScript Math: Exercise-58 with Solution
Volume of a Cone
Write a JavaScript program to calculate the volume of a Cone.
From Wikipedia -
A cone is a three-dimensional geometric shape that tapers smoothly from a flat base (frequently, though not necessarily, circular) to a point called the apex or vertex.
A cone is formed by a set of line segments, half-lines, or lines connecting a common point, the apex, to all of the points on a base that is in a plane that does not contain the apex. Depending on the author, the base may be restricted to be a circle, any one-dimensional quadratic form in the plane, any closed one-dimensional figure, or any of the above plus all the enclosed points.
Sample Data:
Sample Solution:
JavaScript Code:
// Define a function volume_Cone that calculates the volume of a cone given its radius and height.
const volume_Cone = (radius, height) => {
// Check if radius and height are numbers and positive.
is_Number(radius, 'Radius');
is_Number(height, 'Height');
// Return the volume of the cone.
return (Math.PI * radius ** 2 * height / 3.0);
}
// Define a function is_Number that checks if a given value is a number and positive.
const is_Number = (n, n_name = 'number') => {
// If the given value is not a number, throw a TypeError.
if (typeof n !== 'number') {
throw new TypeError('The ' + n_name + ' is not Number type!');
}
// If the given value is negative or not a finite number, throw an Error.
else if (n < 0 || (!Number.isFinite(n))) {
throw new Error('The ' + n_name + ' must be a positive values!');
}
}
// Call the volume_Cone function with valid and invalid arguments and output the results.
console.log(volume_Cone(3.0, 7.0));
console.log(volume_Cone('3.0', 7.0));
console.log(volume_Cone(-3.0, 7.0));
Output:
65.97344572538566 --------------------------------------------------- Uncaught TypeError: The Radius is not Number type! at https://cdpn.io/cpe/boomboom/pen.js?key=pen.js-655e084e-84a8-8f74-b8d6-73c71300d6e2:8 ------------------------------------------------------------------------------------------ Uncaught Error: The Radius must be a positive values! at https://cdpn.io/cpe/boomboom/pen.js?key=pen.js-db99fcf7-e09f-a3d0-3c0d-11b9dabfad0b:10
Flowchart:
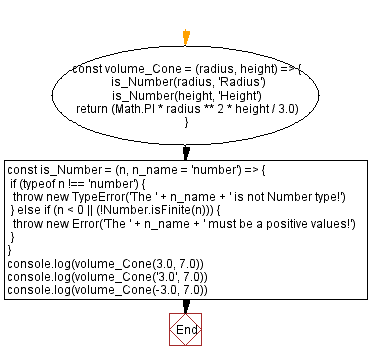
Live Demo:
See the Pen javascript-math-exercise-58 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that calculates the volume of a cone using the formula (1/3)πr²h and validates the input dimensions.
- Write a JavaScript function that computes the volume of a cone and rounds the result to a specified number of decimal places.
- Write a JavaScript function that calculates the volume of a cone and returns an object with both volume and lateral surface area.
- Write a JavaScript function that compares the volume of a cone with that of a cylinder with the same base and height.
Improve this sample solution and post your code through Disqus.
Previous: Volume of a Cube.
Next: Volume of a Pyramid
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.