JavaScript: Sum of the digits of a number
JavaScript Math: Exercise-68 with Solution
Sum of Digits
Write a JavaScript program to calculate the sum of a given number's digits.
In mathematics, the digit sum of a natural number in a given number base is the sum of all its digits. For example, the digit sum of the decimal number 6098 would be 6+0+9+8=23.
Sample Data:
6098 -> 23
-501 -> 6
2546 -> 17
Sample Solution-1:
JavaScript Code:
/**
* Calculates the sum of digits of a given number.
* @param {number} n - The input number.
* @returns {number} - The sum of digits of the input number.
*/
function sum_Of_Digits(n) {
// If the number is negative, convert it to positive
if (n < 0) n = -n;
// Initialize the result variable to store the sum of digits
let result = 0;
// Iterate through each digit of the number and add them to the result
while (n > 0) {
result += n % 10; // Add the last digit to the result
n = Math.floor(n / 10); // Remove the last digit from the number
}
// Return the sum of digits
return result;
}
// Test the function with different input numbers
console.log(sum_Of_Digits(6098)); // Output: 23
console.log(sum_Of_Digits(-501)); // Output: 6
console.log(sum_Of_Digits(2546)); // Output: 17
Output:
23 6 17
Flowchart:
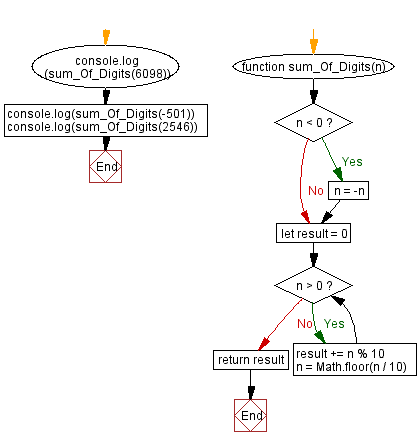
Live Demo:
See the Pen javascript-math-exercise-68 by w3resource (@w3resource) on CodePen.
Sample Solution-2:
JavaScript Code:
/**
* Calculates the sum of digits of a given number recursively.
* @param {number} n - The input number.
* @returns {number} - The sum of digits of the input number.
*/
function sum_Of_Digits(n) {
// If the number is negative, convert it to positive
if (n < 0) n = -n;
// If the number has only one digit, return the number itself
if (n < 10) return n;
// Otherwise, recursively calculate the sum of digits
return (n % 10) + sum_Of_Digits(Math.floor(n / 10));
}
// Test the function with different input numbers
console.log(sum_Of_Digits(6098)); // Output: 23
console.log(sum_Of_Digits(-501)); // Output: 6
console.log(sum_Of_Digits(2546)); // Output: 17
console.log(sum_Of_Digits(10)); // Output: 1
console.log(sum_Of_Digits(5)); // Output: 5
Output:
23 6 17 1 5
Flowchart:
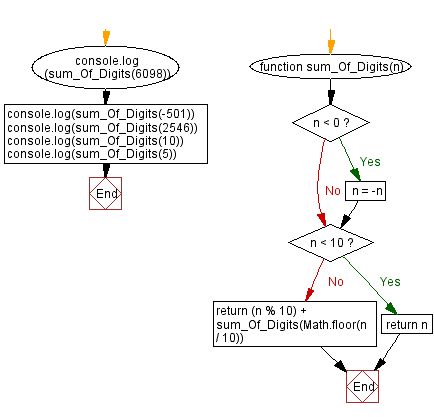
Live Demo:
See the Pen javascript-math-exercise-68-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Sum of a geometric progression.
Next: Find all prime numbers below a given number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/javascript-exercises/javascript-math-exercise-68.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics