JavaScript: Reverse Polish notation in mathematical expression
JavaScript Math: Exercise-70 with Solution
Reverse Polish Notation (RPN)
Write a JavaScript program to apply reverse Polish notation to a given mathematical expression.
From Wikipedia -
Reverse Polish notation (RPN), also known as reverse Łukasiewicz notation, Polish postfix notation or simply postfix notation, is a mathematical notation in which operators follow their operands, in contrast to Polish notation (PN), in which operators precede their operands. It does not need any parentheses as long as each operator has a fixed number of operands. The description "Polish" refers to the nationality of logician Jan Łukasiewicz, who invented Polish notation in 1924.
Sample Data:
"5 8 *" -> 40
"5 6 + 2 *" -> 22
"5 3 4 * +" -> 17
Sample Solution:
JavaScript Code:
/**
* Performs Reverse Polish Notation (RPN) calculation on the given mathematical expression.
* @param {string} math_expr - The mathematical expression in Reverse Polish Notation.
* @returns {number} - The result of the calculation.
*/
const RPN_calculation = (math_expr) => {
// Define operators and their corresponding functions
const operators = {
'+': (x, y) => x + y,
'-': (x, y) => x - y,
'*': (x, y) => x * y,
'/': (x, y) => y / x
};
// Split the mathematical expression into parts
const expr_part = math_expr.split(' ');
// Initialize an array to store intermediate results
const data = [];
// Iterate over each part of the expression
expr_part.forEach((expr_part) => {
// Check if the current part is an operator
const operator = operators[expr_part];
if (typeof operator === 'function') {
// If it's an operator, pop the last two values from 'data', apply the operator, and push the result back
const x = data.pop();
const y = data.pop();
const result = operator(x, y);
data.push(result);
} else {
// If it's not an operator, parse it as a float and push it to 'data'
data.push(parseFloat(expr_part));
}
});
// Return the final result
return data.pop();
};
// Test the function with different RPN expressions
console.log(RPN_calculation("5 8 *")); // Output: 40
console.log(RPN_calculation("5 6 + 2 *")); // Output: 22
console.log(RPN_calculation("5 3 4 * +")); // Output: 17
Output:
40 22 17
Flowchart:
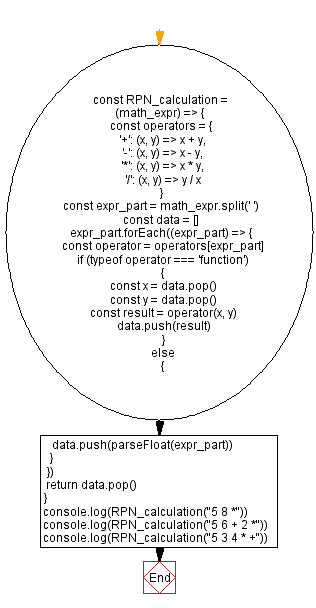
Live Demo:
See the Pen javascript-math-exercise-70 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that evaluates a mathematical expression written in Reverse Polish Notation using a stack-based approach.
- Write a JavaScript function that converts an infix expression to Reverse Polish Notation and then evaluates it.
- Write a JavaScript function that handles error cases such as insufficient operands and invalid operators in an RPN expression.
- Write a JavaScript function that supports both integer and floating-point arithmetic when evaluating an RPN expression.
Improve this sample solution and post your code through Disqus.
Previous: Find all prime numbers below a given number.
Next: All prime factors of a given number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.