JavaScript: Two positive integers as string, return their sum
JavaScript Math: Exercise-77 with Solution
Sum Two Non-Negative Integers (Strings)
Write a JavaScript program to compute the sum of two non-negative integers num1 and num2 represented by strings.
Test Data:
("123", "123") -> 246
("45", "100") -> 145
Sample Solution:
JavaScript Code:
/**
* Function to perform addition of two large numbers represented as strings.
* @param {string} n1 - The first number as a string.
* @param {string} n2 - The second number as a string.
* @returns {string} - The sum of the two numbers as a string.
*/
function test(n1, n2) {
// Initialize an empty string to store the result
var str = '';
// Initialize the carry to 0
var carry = 0;
// Initialize the index for the first number
var num1 = n1.length - 1;
// Initialize the index for the second number
var num2 = n2.length - 1;
// Initialize a variable to store the sum of two digits
var sum_of_two;
// Loop until both numbers are exhausted
while (num1 >= 0 || num2 >= 0) {
// Calculate the sum of two digits from each number and add the carry
sum_of_two = (parseInt(n1[num1]) || 0) + (parseInt(n2[num2]) || 0)
sum_of_two += carry ? 1 : 0;
// If the sum is greater than 9, set the carry and append the unit digit to the result
if (sum_of_two > 9) {
carry = 1;
str = sum_of_two % 10 + str;
} else {
// If the sum is less than or equal to 9, append the sum to the result
str = sum_of_two + str;
// Reset the carry to 0
carry = 0;
}
// Move to the next digits of both numbers
num1--;
num2--;
}
// If there is still a carry after the loop, append it to the result
if (carry) {
str = 1 + str;
}
// Return the final result
return str;
}
// Test cases
console.log(test("123", "123"));
console.log(test("45", "100"));
Output:
246 145
Flowchart:
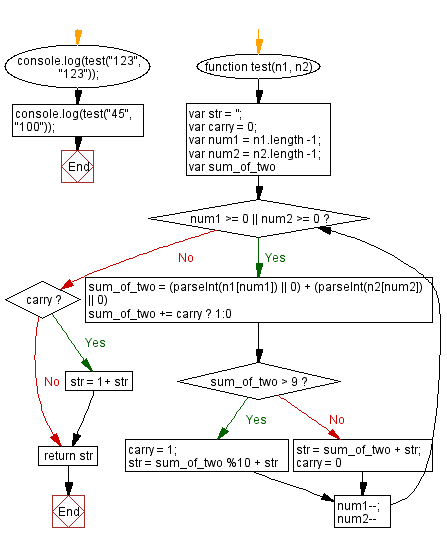
Live Demo:
See the Pen javascript-math-exercise-77 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that adds two non-negative integers represented as strings and returns their sum as a string.
- Write a JavaScript function that simulates manual addition of two numeric strings digit by digit from right to left.
- Write a JavaScript function that handles carry-over operations when adding two string-represented numbers.
- Write a JavaScript function that validates input strings for non-numeric characters before performing addition.
Improve this sample solution and post your code through Disqus.
Previous: Subtract without arithmetic operators.
Next: Nth Tetrahedral Number
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.