JavaScript: Multiply every digit of a number three times
JavaScript Math: Exercise-81 with Solution
Multiply Digits of Number by Three
Write a JavaScript program to multiply every digit of a number three times.
Test Data:
(11) -> 11
(66) -> 216216
(336) -> 2727216
(444) -> 646464
(1151) -> 111251
Sample Solution:
JavaScript Code:
/**
* Function to multiply every digit of a number three times and concatenate the results.
* @param {number} n - The input number.
* @returns {number} - The result of multiplying every digit of the input number three times.
*/
function test(n) {
// Convert the number to a string, map each digit to its cube, and join them as a string
return +[...String(n)].map(x => x * x * x).join('');
}
// Test cases
// Assign value to n
n = 11;
// Print the value of n
console.log("n = " + n);
// Multiply every digit of the said number three times
console.log("Multiply every digit of the said number three times: " + test(n));
// Repeat the above steps for different values of n
n = 66;
console.log("n = " + n);
console.log("Multiply every digit of the said number three times: " + test(n));
n = 336;
console.log("n = " + n);
console.log("Multiply every digit of the said number three times: " + test(n));
n = 444;
console.log("n = " + n);
console.log("Multiply every digit of the said number three times: " + test(n));
n = 1151;
console.log("n = " + n);
console.log("Multiply every digit of the said number three times: " + test(n));
Output:
n = 11 Multiply every digit of the said number three times: 11 n = 66 Multiply every digit of the said number three times: 216216 n = 336 Multiply every digit of the said number three times: 2727216 n = 444 Multiply every digit of the said number three times: 646464 n = 1151 Multiply every digit of the said number three times: 111251
Flowchart:
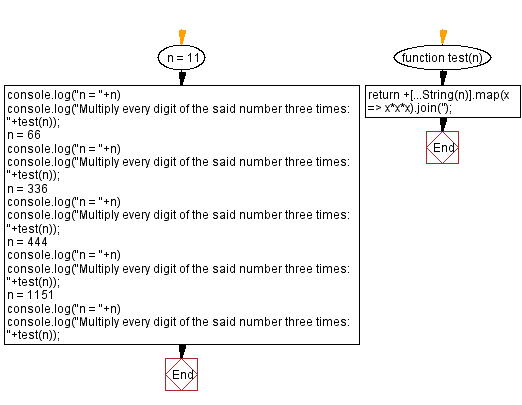
Live Demo:
See the Pen javascript-math-exercise-81 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that multiplies every digit of a number by three and concatenates the results into a new number.
- Write a JavaScript function that converts a number to a string, multiplies each digit by three, and returns the concatenated result.
- Write a JavaScript function that validates the input number and processes each digit individually to perform the multiplication.
- Write a JavaScript function that iterates over a numeric string and constructs a new string by tripling each digit.
Go to:
PREV : Check Repdigit Number.
NEXT : Mean of Digits in a Number.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.