JavaScript: Even, odd numbers and their sum in an array
JavaScript Math: Exercise-94 with Solution
Sum and Count of Even and Odd Numbers
Write a JavaScript program to calculate the sum and count of even and odd numbers in an array.
Test Data:
([1,2,3,4,5,6,7]) -> 3,12, 4,16
([2,3,5,1,2,0,3,4,2,3,4)] -> 6,14, 5,15
Sample Solution:
JavaScript Code:
/**
* Function to count the number of even and odd numbers and their sums in an array.
* @param {number[]} nums - The array of numbers to be processed.
* @returns {number[]} - An array containing the count and sum of even and odd numbers, respectively.
*/
function test(nums) {
let even = 0, odd = 0;
nums.forEach(number => {
if (number % 2 === 0)
even++;
else
odd++;
});
return [
even,
nums.reduce((s, v) => (!(v % 2) ? s + v : s), 0),
odd,
nums.reduce((s, v) => (v % 2 ? s + v : s), 0)
];
}
// Test cases
let nums = [1, 2, 3, 4, 5, 6, 7];
console.log("Original array: " + nums);
let result = test(nums);
console.log("Number of even numbers and their sum: " + result[0] + "," + result[1]);
console.log("Number of odd numbers and their sum: " + result[2] + "," + result[3]);
nums = [2, 3, 5, 1, 2, 0, 3, 4, 2, 3, 4];
console.log("Original array: " + nums);
result = test(nums);
console.log("Number of even numbers and their sum: " + result[0] + "," + result[1]);
console.log("Number of odd numbers and their sum: " + result[2] + "," + result[3]);
Output:
Original array: 1,2,3,4,5,6,7 Number of even numbers and their sum: 3,12 Number of odd numbers and their sum: 4,16 Original array: 2,3,5,1,2,0,3,4,2,3,4 Number of even numbers and their sum: 6,14 Number of odd numbers and their sum: 5,15
Flowchart:
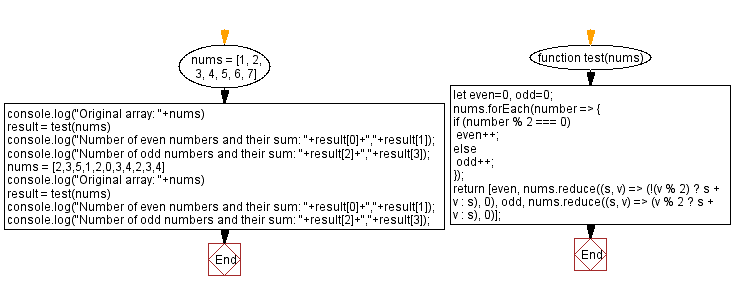
Live Demo:
See the Pen javascript-math-exercise-94 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that iterates through an array and separately calculates the sum and count of even numbers.
- Write a JavaScript function that uses the reduce() method to compute the sum and count of odd numbers in an array.
- Write a JavaScript function that returns an object with properties evenCount, evenSum, oddCount, and oddSum after processing an array.
- Write a JavaScript function that validates the input array and handles cases where one type (even/odd) is absent.
Improve this sample solution and post your code through Disqus.
Previous: Check downward trend, array of integers.
Next: Last and middle character from a string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.