JavaScript: Add digits until there is only one digit
JavaScript Math: Exercise-99 with Solution
Sum Digits Until One Digit
Write a JavaScript program to add repeatedly all the digits of a given non-negative number until the result has only one digit.
For example:
Input: 39
Output: 3
Explanation: The formula is like: 3 + 9 = 12, 1 + 2 = 3.
Visual Presentation:
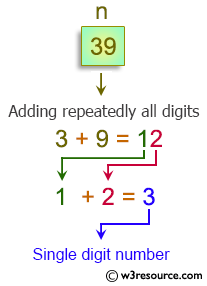
Test Data:
(47) -> 2
(9) -> 9
Sample Solution-1:
JavaScript Code:
/**
* Function to add the digits of a number until there is only one digit left.
* @param {number} n - The number to process.
* @returns {number} - The result of adding digits until only one digit remains.
*/
function test(n) {
if (isNaN(n) || n === 0) return 0; // Return 0 if input is not a number or is 0
total = n; // Initialize total with the input number
while (n >= 10) { // Loop until the number becomes a single digit
total = 0; // Reset total
while (n != 0) { // Loop to add digits of the number
total += Math.floor(n % 10); // Add the last digit to total
n = Math.floor(n / 10); // Remove the last digit
}
n = total; // Update n with the new total
}
return total; // Return the final total
};
// Test cases
n = 49;
console.log("n = " +n);
console.log("Add digits of the said number until there is only one digit: "+test(n));
n = 9;
console.log("Original text: " +n);
console.log("Add digits of the said number until there is only one digit: "+test(n));
Output:
n = 49 Add digits of the said number until there is only one digit: 4 Original text: 9 Add digits of the said number until there is only one digit: 9
Flowchart:
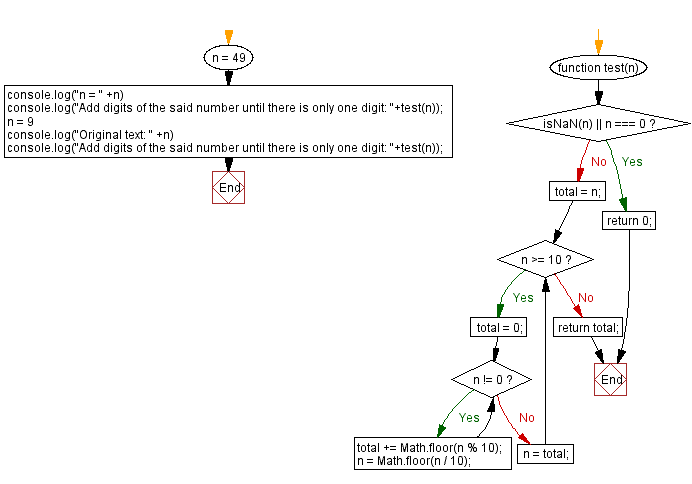
Live Demo:
See the Pen javascript-math-exercise-98-1 by w3resource (@w3resource) on CodePen.
Sample Solution-2:
JavaScript Code:
/**
* Function to add the digits of a number until there is only one digit left.
* @param {number} n - The number to process.
* @returns {number} - The result of adding digits until only one digit remains.
*/
function test(n) {
if (isNaN(n) || n === 0) return 0; // Return 0 if input is not a number or is 0
if (n < 10) return n; // Return the number itself if it is already a single digit
return n % 9 === 0 ? 9 : n % 9; // If n is divisible by 9, return 9; otherwise, return the remainder of n divided by 9
};
// Test cases
n = 49;
console.log("n = " +n);
console.log("Add digits of the said number until there is only one digit: "+test(n));
n = 9;
console.log("Original text: " +n);
console.log("Add digits of the said number until there is only one digit: "+test(n));
Output:
n = 49 Add digits of the said number until there is only one digit: 4 Original text: 9 Add digits of the said number until there is only one digit: 9
Flowchart:
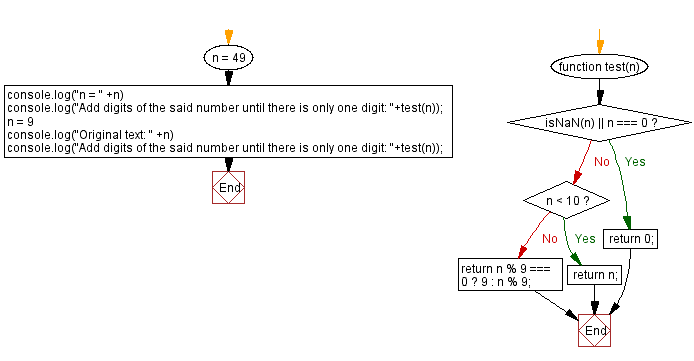
Live Demo:
See the Pen javascript-math-exercise-99-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that repeatedly sums the digits of a number until a single digit remains, returning that digit.
- Write a JavaScript function that implements the digital root algorithm using a loop.
- Write a JavaScript function that uses recursion to reduce a number to its digital root.
- Write a JavaScript function that validates non-negative input before performing repeated digit summation.
Improve this sample solution and post your code through Disqus.
Previous: Column number that relates to a column title.
Next: Check a given number is an ugly number or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.