JavaScript: Get a copy of the object where the keys have become the values and the values the keys
JavaScript Object: Exercise-16 with Solution
Swap Keys and Values
Write a JavaScript function to get a copy of the object where the keys become the values and the values are the keys.
Sample Solution:
JavaScript Code:
function invert_key_value(obj) {
var result = {};
var keys = _keys(obj);
for (var i = 0, length = keys.length; i < length; i++) {
result[obj[keys[i]]] = keys[i];
}
return result;
}
function _keys(obj)
{
if (!isObject(obj)) return [];
if (Object.keys) return Object.keys(obj);
var keys = [];
for (var key in obj) if (_.has(obj, key)) keys.push(key);
return keys;
}
function isObject(obj)
{
var type = typeof obj;
return type === 'function' || type === 'object' && !!obj;
}
console.log(invert_key_value({red: "#FF0000", green: "#00FF00", white: "#FFFFFF"}));
Output:
{"#FF0000":"red","#00FF00":"green","#FFFFFF":"white"}
Flowchart:
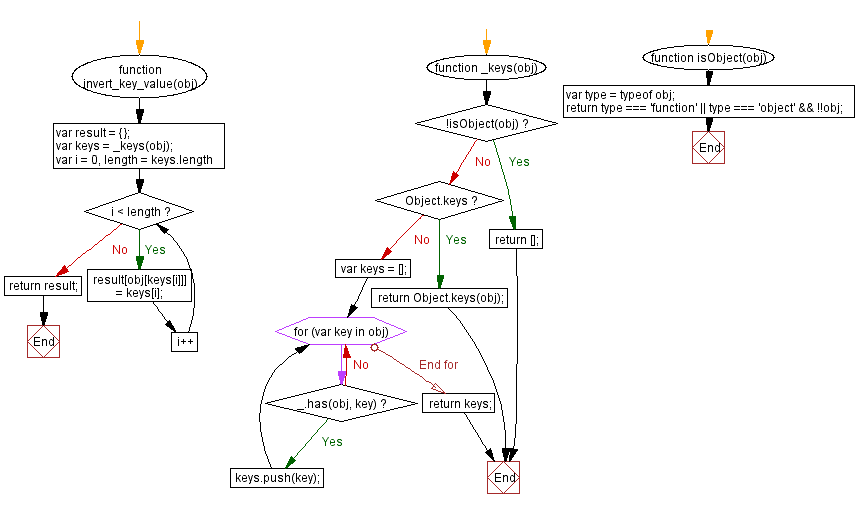
Live Demo:
See the Pen javascript-object-exercise-16 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that creates a new object by swapping keys and values of the original object.
- Write a JavaScript function that converts an object to key-value pairs, swaps each pair, and rebuilds the object.
- Write a JavaScript function that handles duplicate values by storing them in an array under the new key.
- Write a JavaScript function that validates the input and returns an error if the object contains non-primitive values.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript function to convert an object into a list of '[key, value]' pairs.
Next: Write a JavaScript function to check if an object contains given property.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.