JavaScript: Compute the sum of an array of integers
JavaScript Function: Exercise-4 with Solution
Sum of Array Elements
Write a JavaScript program to compute the sum of an array of integers.
Example : var array = [1, 2, 3, 4, 5, 6]
Expected Output : 21
Visual Presentation:
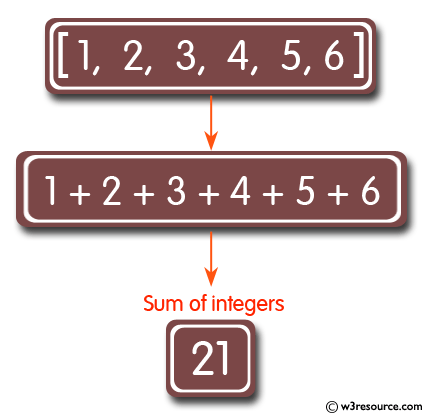
Sample Solution-1:
JavaScript Code:
// Function to calculate the sum of elements in an array using recursion.
var array_sum = function(my_array) {
// Base case: if the array has only one element, return that element.
if (my_array.length === 1) {
return my_array[0];
}
else {
// Recursive case: pop the last element and add it to the sum of the remaining elements.
return my_array.pop() + array_sum(my_array);
}
};
// Example usage: Calculate and print the sum of elements in the array [1, 2, 3, 4, 5, 6].
console.log(array_sum([1, 2, 3, 4, 5, 6]));
Output:
21
Flowchart:
Live Demo:
See the Pen javascript-recursion-function-exercise-4 by w3resource (@w3resource) on CodePen.
Sample Solution-2:
JavaScript Code:
// Function to calculate the sum of elements in an array using recursion.
function arraySumRecursive(arr, index = 0) {
// Base case: if the index exceeds the array length, return 0.
if (index >= arr.length) {
return 0;
} else {
// Recursive case: add the current element to the sum of the rest of the array.
return arr[index] + arraySumRecursive(arr, index + 1);
}
}
// Example usage: Calculate and print the sum of elements in the array [1, 2, 3, 4, 5, 6].
console.log(arraySumRecursive([1, 2, 3, 4, 5, 6]));
Output:
21
Flowchart:
For more Practice: Solve these Related Problems:
- Write a JavaScript function that recursively computes the sum of an array of integers without using loops.
- Write a JavaScript function that sums all the elements in an array and returns zero for an empty array.
- Write a JavaScript function that recursively computes the sum of an array while skipping non-numeric values.
- Write a JavaScript function that uses a divide and conquer approach recursively to compute the sum of array elements.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript program to get the integers in range (x, y).
Next: Write a JavaScript program to compute the exponent of a number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.