JavaScript: Check whether the pattern of an e-mail address matches a specific format
JavaScript validation with regular expression: Exercise-3 with Solution
Email Pattern
Write a pattern that matches e-mail addresses.
The personal information part contains the following ASCII characters.
Sample Solution: 1
JavaScript Code:
function valid_email(str)
{
var mailformat = /^\w+([\.-]?\w+)*@\w+([\.-]?\w+)*(\.\w{2,3})+$/;
if(mailformat.test(str))
{
console.log("Valid email address!");
}
else
{
console.log("You have entered an invalid email address!");
}
}
valid_email('[email protected]');
Output:
Valid email address!
Flowchart:
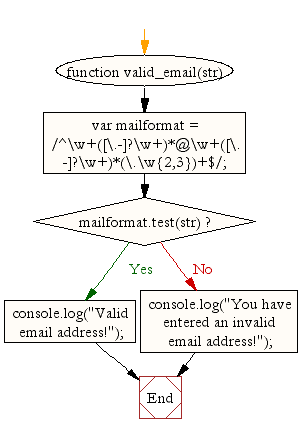
Sample Solution:- 2
JavaScript Code:
function is_email(str)
{
// Scott Gonzalez: Email address validation
regexp = /^((([a-z]|\d|[!#\$%&'\*\+\-\/=\?\^_`{\|}~]|[\u00A0-\uD7FF\uF900-\uFDCF\uFDF0-\uFFEF])+(\.([a-z]|\d|[!#\$%&'\*\+\-\/=\?\^_`{\|}~]|[\u00A0-\uD7FF\uF900-\uFDCF\uFDF0-\uFFEF])+)*)|((\x22)((((\x20|\x09)*(\x0d\x0a))?(\x20|\x09)+)?(([\x01-\x08\x0b\x0c\x0e-\x1f\x7f]|\x21|[\x23-\x5b]|[\x5d-\x7e]|[\u00A0-\uD7FF\uF900-\uFDCF\uFDF0-\uFFEF])|(\\([\x01-\x09\x0b\x0c\x0d-\x7f]|[\u00A0-\uD7FF\uF900-\uFDCF\uFDF0-\uFFEF]))))*(((\x20|\x09)*(\x0d\x0a))?(\x20|\x09)+)?(\x22)))@((([a-z]|\d|[\u00A0-\uD7FF\uF900-\uFDCF\uFDF0-\uFFEF])|(([a-z]|\d|[\u00A0-\uD7FF\uF900-\uFDCF\uFDF0-\uFFEF])([a-z]|\d|-|\.|_|~|[\u00A0-\uD7FF\uF900-\uFDCF\uFDF0-\uFFEF])*([a-z]|\d|[\u00A0-\uD7FF\uF900-\uFDCF\uFDF0-\uFFEF])))\.)+(([a-z]|[\u00A0-\uD7FF\uF900-\uFDCF\uFDF0-\uFFEF])|(([a-z]|[\u00A0-\uD7FF\uF900-\uFDCF\uFDF0-\uFFEF])([a-z]|\d|-|\.|_|~|[\u00A0-\uD7FF\uF900-\uFDCF\uFDF0-\uFFEF])*([a-z]|[\u00A0-\uD7FF\uF900-\uFDCF\uFDF0-\uFFEF])))$/i;
if (regexp.test(str))
{
return true;
}
else
{
return false;
}
}
console.log(is_email("[email protected]"));
console.log(is_email("[email protected] "));
Live Demo:
See the Pen javascript-regexp-exercise-3 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript regular expression that matches a valid e-mail address following the given ASCII character rules.
- Write a JavaScript function that uses a regex pattern to extract the username and domain parts of an e-mail address.
- Write a JavaScript function that tests multiple e-mail addresses against your regex and returns those that match the criteria.
- Write a JavaScript function that refines a regex to disallow consecutive dots and ensure the personal part does not start or end with a dot.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript program to check a credit card number ( format 9999-9999-9999-9999 ).
Next: Write a JavaScript program to search a date within a string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.