JavaScript: Count number of words in string
JavaScript validation with regular expression: Exercise-6 with Solution
Count Words in String
Write a JavaScript program to count number of words in string.
The script will be used to:
- Remove white-space from start and end position.- Convert 2 or more spaces to 1.
- Exclude newline with a start spacing.
Visual Presentation:
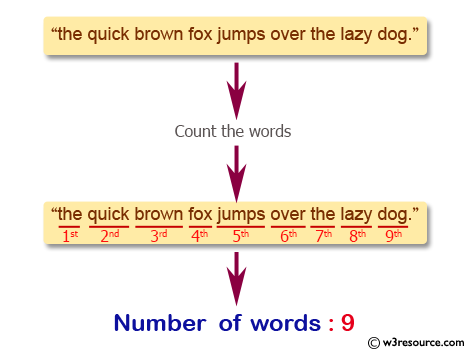
Sample Solution:
HTML Code:
<!DOCTYPE html>
<html>
<head>
<meta charset=utf-8 />
<title>Count number of words in a string</title>
<style type="text/css">
body {margin-top: 40px;}
</style>
</head>
<body>
<textarea id="InputText" cols="30" rows="4">The quick brown fox jumps over the lazy dog.</textarea>
<br>
<input type="button" name="Convert" value="No. of Words" onClick="count_words();">
<input id = "noofwords" type="text" value="" size="6">
</body>
</html>
JavaScript Code:
function count_words()
{
str1= document.getElementById("InputText").value;
//exclude start and end white-space
str1 = str1.replace(/(^\s*)|(\s*$)/gi,"");
//convert 2 or more spaces to 1
str1 = str1.replace(/[ ]{2,}/gi," ");
// exclude newline with a start spacing
str1 = str1.replace(/\n /,"\n");
document.getElementById("noofwords").value = str1.split(' ').length;
}
Output:
The quick brown fox jumps over the lazy dog. 9
Live Demo:
See the Pen javascript-regexp-exercise-6 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that counts the number of words in a string by first trimming and then splitting by whitespace.
- Write a JavaScript function that collapses multiple spaces into one and returns the accurate word count.
- Write a JavaScript function that excludes punctuation from words before counting the total number.
- Write a JavaScript function that accepts a string with newline characters and counts words correctly across lines.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript program that works as a trim function (string) using regular expression.
Next: Write a JavaScript function to check whether a given value is IP value or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.