JavaScript: Make a string uncamelize
JavaScript String: Exercise-12 with Solution
Uncamelize String
Write a JavaScript function to uncommelize a string.
Test Data:
console.log(uncamelize('helloWorld'));
console.log(uncamelize('helloWorld','-'));
console.log(uncamelize('helloWorld','_'));
"hello world"
"hello-world"
"hello_world"
Visual Presentation:
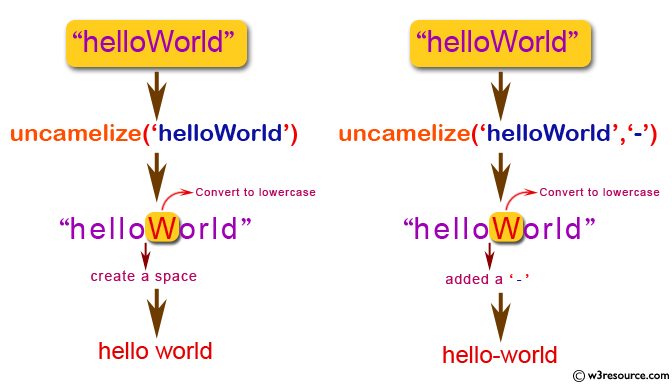
Sample Solution:-
JavaScript Code:
// Define a function called uncamelize that takes two parameters: a string (str) and an optional separator
function uncamelize(str, separator) {
// Assume default separator is a single space.
if(typeof(separator) == "undefined") {
separator = " ";
}
// Replace all capital letters by separator followed by lowercase one
var str = str.replace(/[A-Z]/g, function (letter)
{
return separator + letter.toLowerCase();
});
// Remove first separator
return str.replace("/^" + separator + "/", '');
}
// Test the function with different inputs and separators and print the results to the console
console.log(uncamelize('helloWorld'));
console.log(uncamelize('helloWorld','-'));
console.log(uncamelize('helloWorld','_'));
Output:
hello world hello-world hello_world
Explanation:
In the exercise above,
The code defines a function called "uncamelize()" which takes a string 'str' and an optional 'separator' parameter. If the 'separator' is not provided, it defaults to a single space.
- The function replaces all capital letters in the string with the separator followed by the lowercase version of the letter.
- Then it removes the first occurrence of the separator from the beginning of the string.
- Finally, it returns the modified string.
Flowchart:
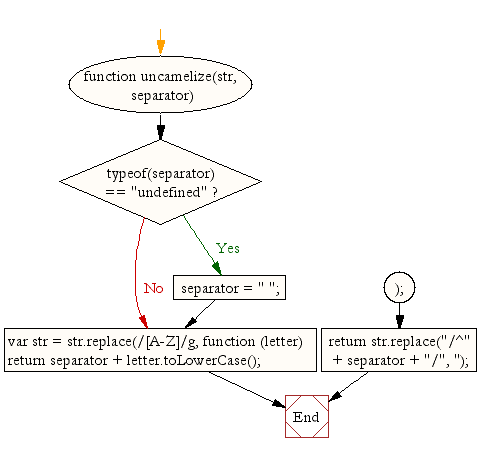
Live Demo:
See the Pen JavaScript Make a string uncamelize - string-ex-12 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that inserts a space (or specified character) before each uppercase letter in a camelCase string.
- Write a JavaScript function that converts a camelCase string into a lowercase, space-separated string.
- Write a JavaScript function that handles edge cases where the input is empty or already uncamelized.
- Write a JavaScript function that uses regular expressions to detect uppercase letters and insert delimiters accordingly.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to convert a string into camel case.
Next: Write a JavaScript function to concatenates a given string n times (default is 1).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.