JavaScript: Alphabetize a given string
JavaScript String: Exercise-25 with Solution
Alphabetize String
Write a JavaScript function to alphabetize a given string.
Alphabetize string : An individual string can be alphabetized. This rearranges the letters so they are sorted A to Z.
Test Data:
console.log(alphabetize_string('United States'));
Output:
"SUadeeinsttt"
Visual Presentation:
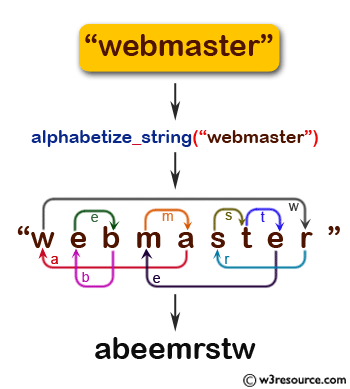
Sample Solution:
JavaScript Code:
// Function to alphabetize a string
function alphabetize_string(str)
{
// Split the string into an array of characters, sort them alphabetically, then join them back into a string
return str.split('').sort().join('').trim();
}
// Output the result of alphabetizing the string 'United States'
console.log(alphabetize_string('United States'));
Output:
SUadeeinsttt
Explanation:
The above JavaScript code defines a function called "alphabetize_string()" that takes a string ('str') as input and returns the string with its characters sorted alphabetically. Here's a breakdown of what each part does:
- str.split(''): Splits the input string into an array of characters.
- .sort(): Sorts the array of characters alphabetically.
- .join(''): Joins the sorted array back into a string.
- .trim(): Removes any leading or trailing whitespace from the resulting string.
- return: Returns the alphabetized string.
Flowchart:
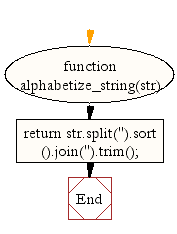
Live Demo:
See the Pen JavaScript Alphabetize a given string - string-ex-25 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that rearranges the letters of a string in alphabetical order.
- Write a JavaScript function that converts a string to lowercase, sorts its characters, and returns the new string.
- Write a JavaScript function that handles strings with spaces and punctuation by ignoring non-letter characters.
- Write a JavaScript function that compares the alphabetized result of a string with a pre-sorted expected string.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to truncate a string to a certain number of words.
Next: Write a JavaScript function to remove the first occurrence of a given 'search string' from a string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.