JavaScript: Remove non-word characters
JavaScript String : Exercise-33 with Solution
Remove Non-Word Characters
Write a JavaScript function to remove non-word characters.
Test Data :
console.log(remove_non_word('PHP ~!@#$%^&*()+`-={}[]|\\:";\'/?><., MySQL'));
"PHP - MySQL"
Visual Presentation:
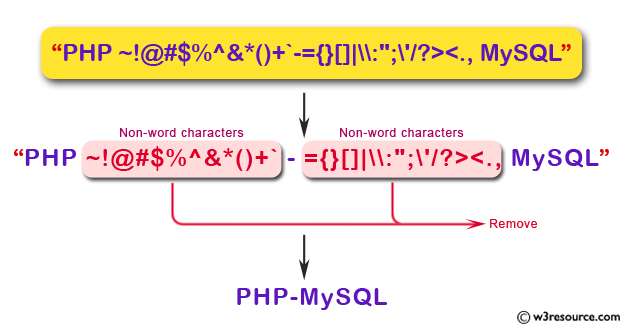
Sample Solution:
JavaScript Code :
// Define a function named remove_non_word which takes a string parameter str
function remove_non_word (str) {
// Check if the input string is null or empty
if ((str===null) || (str===''))
// If the input string is null or empty, return false
return false;
else
// If the input string is not null or empty, convert it to a string type
str = str.toString();
// Define a regular expression pattern to match non-word characters
var PATTERN = /[^\x20\x2D0-9A-Z\x5Fa-z\xC0-\xD6\xD8-\xF6\xF8-\xFF]/g;
// Use the replace method with the defined pattern to remove non-word characters from the string
return str.replace(PATTERN, '');
}
// Call the remove_non_word function with a test string and log the result to the console
console.log(remove_non_word('PHP ~!@#$%^&*()+`-={}[]|\\:";\'/?><., MySQL'));
Output:
PHP - MySQL
Explanation:
In the exercise above,
- The function "remove_non_word()" takes a string 'str' as input.
- It checks if the input string is null or empty. If so, it returns false.
- If the input string is not null or empty, it converts it to a string type.
- A regular expression pattern "PATTERN" is defined to match non-word characters.
- The "replace()" method is used to remove non-word characters from the string using the defined pattern.
- Finally, the function returns the modified string with non-word characters removed.
Flowchart :
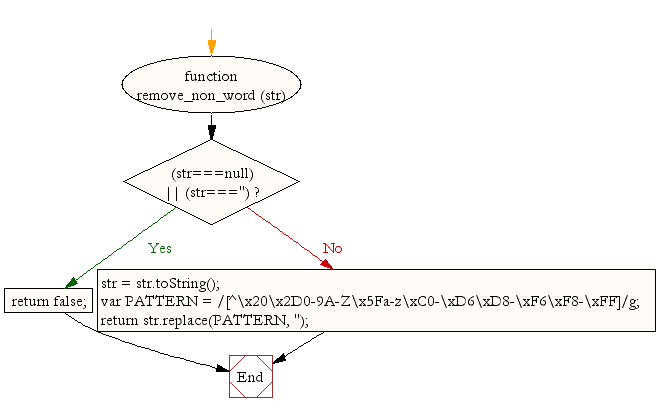
Live Demo :
See the Pen JavaScript Remove non-word characters-string-ex-33 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that removes all non-word characters (anything other than letters, digits, and underscores) from a string.
- Write a JavaScript function that uses a regular expression to filter out special characters while preserving spaces.
- Write a JavaScript function that validates the input string and returns only alphanumeric characters and spaces.
- Write a JavaScript function that strips punctuation and symbols from a string to leave only words.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to remove non-printable ASCII chars.
Next: Write a JavaScript function to convert a string to title case.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.