JavaScript Exercises: Count number of nodes in a Doubly Linked Lists
JavaScript Data Structures: Exercise-2 with Solution
Create a DLL of n nodes and count the number of nodes
Write a JavaScript program to create a Doubly Linked Lists of n nodes and count the number of nodes.
Sample Solution:
JavaScript Code:
// Define a class for creating nodes of a doubly linked list
class Node {
constructor(value) {
this.value = value; // Store the value of the node
this.next = null; // Pointer to the next node in the list, initially set to null
this.previous = null; // Pointer to the previous node in the list, initially set to null
}
}
// Define a class for creating a doubly linked list
class DoublyLinkedList {
constructor(value) {
// Initialize the head node with the given value and no next or previous node
this.head = {
value: value, // Store the value of the head node
next: null, // Pointer to the next node in the list, initially set to null
previous: null // Pointer to the previous node in the list, initially set to null
};
this.length = 0; // Initialize the length of the list to 0
this.tail = this.head; // Set the tail node to the head node initially
}
// Method to add a new node at the end of the list
add(newNode) {
// Check if the head is null, indicating an empty list
if (this.head === null) {
this.head = newNode; // Set the head to the new node
this.tail = newNode; // Set the tail to the new node
} else {
newNode.previous = this.tail; // Set the previous pointer of the new node to the current tail
this.tail.next = newNode; // Set the next pointer of the current tail to the new node
this.tail = newNode; // Update the tail to the new node
}
this.length++; // Increment the length of the list
}
// Method to return the size of the doubly linked list
size() {
return this.length; // Return the length of the list
}
// Method to print the values of the nodes in the list
printList() {
let current = this.head; // Start from the head of the list
let result = []; // Array to store the values of the nodes
while (current !== null) { // Iterate through the list until reaching the end
result.push(current.value); // Push the value of the current node to the array
current = current.next; // Move to the next node
}
console.log(result.join(' ')); // Log the values of the nodes separated by space
return this; // Return the DoublyLinkedList object for chaining
}
}
// Create a new instance of the DoublyLinkedList class
let numList = new DoublyLinkedList();
// Add nodes to the list
numList.add(new Node(2));
numList.add(new Node(3));
numList.add(new Node(5));
numList.add(new Node(6));
numList.add(new Node(8));
// Display the original doubly linked list
console.log("Original Doubly Linked Lists:")
numList.printList();
// Get the size of the list and display it
let linkedlist_size = numList.size();
console.log("Size of the said Doubly Linked lists: "+linkedlist_size);
Output:
Original Doubly Linked Lists: 2 3 5 6 8 Size of the said Doubly Linked lists: 5
Flowchart:
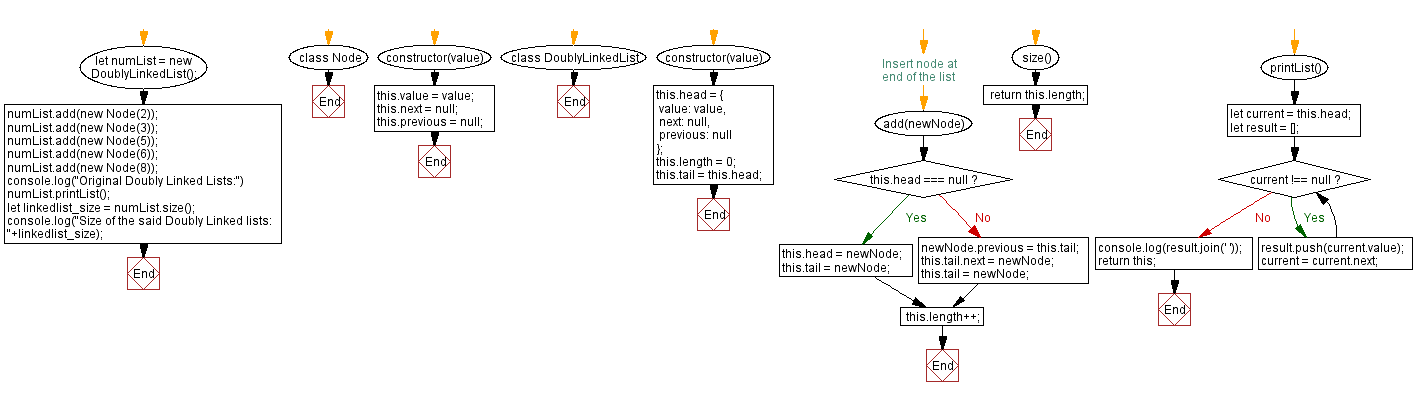
Live Demo:
See the Pen javascript-doubly-linked-list-exercise-2 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Linked List Previous: Create and display doubly linked list.
Linked List Next: Check whether a Doubly Linked Lists is empty or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/javascript-exercises/linkedlist/javascript-doubly-linked-list-exercise-2.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics