JavaScript Sorting Algorithm: Sort an array of objects, ordered by properties and orders
JavaScript Sorting Algorithm: Exercise-16 with Solution
Sort Objects by Properties
Write a JavaScript program to sort an array of objects, ordered by properties and orders.
- Uses Array.prototype.sort(), Array.prototype.reduce() on the props array with a default value of 0.
- Use array destructuring to swap the properties position depending on the order supplied.
- If no orders array is supplied, sort by 'asc' by default.
Sample Solution:
JavaScript Code:
const orderBy = (arr, props, orders) =>
[...arr].sort((a, b) =>
props.reduce((acc, prop, i) => {
if (acc === 0) {
const [p1, p2] =
orders && orders[i] === 'desc'
? [b[prop], a[prop]]
: [a[prop], b[prop]];
acc = p1 > p2 ? 1 : p1 < p2 ? -1 : 0;
}
return acc;
}, 0)
);
const users = [
{ name: 'fred', age: 48 },
{ name: 'barney', age: 36 },
{ name: 'fred', age: 40 },
];
console.log(orderBy(users, ['name', 'age'], ['asc', 'desc']));
console.log(orderBy(users, ['name', 'age']));
Sample Output:
[{"name":"barney","age":36},{"name":"fred","age":48},{"name":"fred","age":40}] [{"name":"barney","age":36},{"name":"fred","age":40},{"name":"fred","age":48}]
Flowchart:
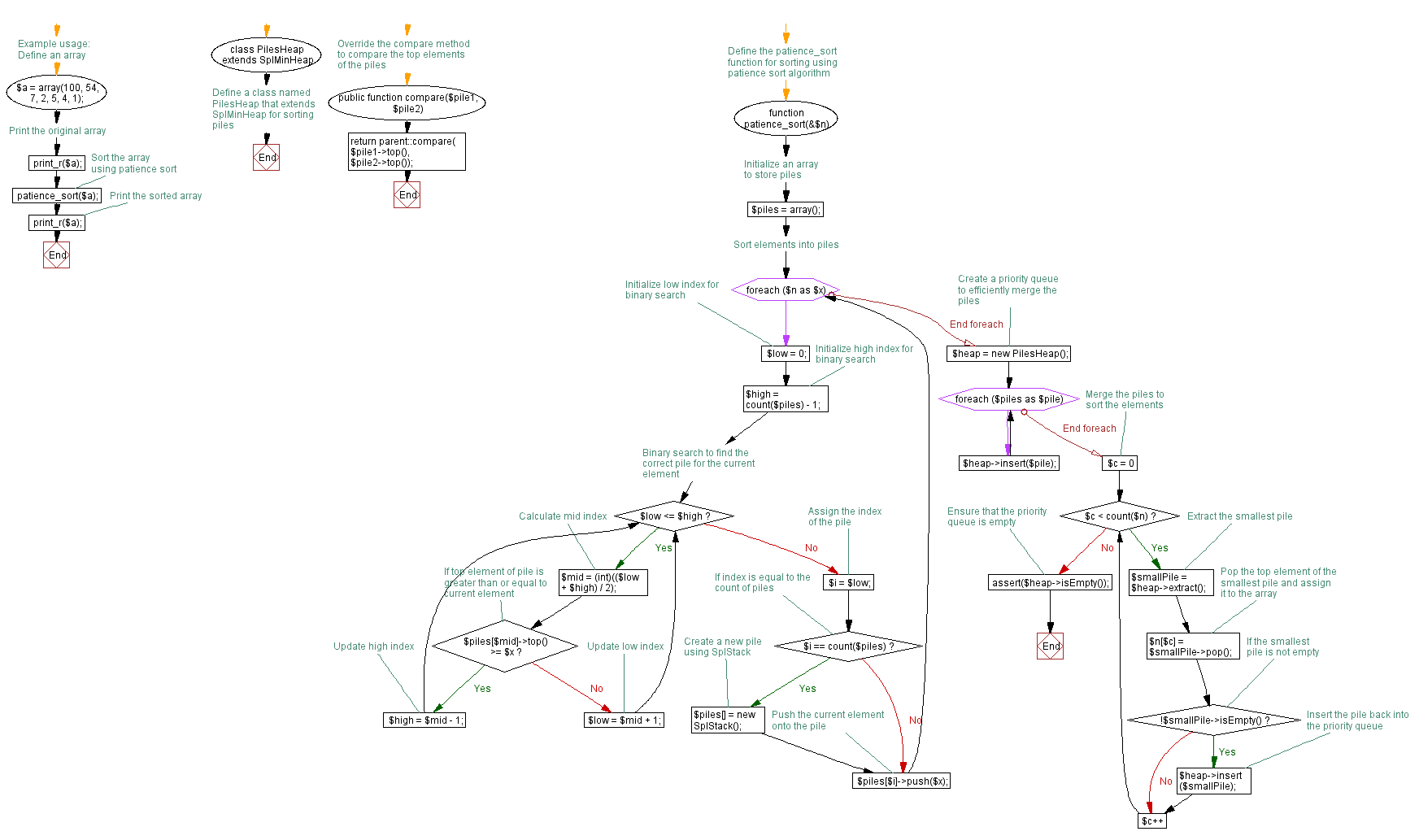
Live Demo:
See the Pen javascript-common-editor by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that sorts an array of objects by a specified property in ascending order.
- Write a JavaScript function that sorts objects first by one property, then by a secondary property if values are equal.
- Write a JavaScript function that sorts an array of objects using a custom comparator and logs the sorted result.
- Write a JavaScript function that handles objects with missing properties by placing them at the end of the sorted array.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to sort an array of numbers, using the bucket sort algorithm.
Next: Write a JavaScript program to find the highest index at which a value should be inserted into an array in order to maintain its sort order, based on a provided iterator function.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.