JavaScript Sorting Algorithm: Sorted Index
JavaScript Sorting Algorithm: Exercise-22 with Solution
Find Lowest Insert Index (Array)
Write a JavaScript program to find the lowest index at which a value should be inserted into an array to maintain its sorting order.
- Loosely check if the array is sorted in descending order.
- Use Array.prototype.findIndex() to find the appropriate index where the element should be inserted.
Sample Solution:
JavaScript Code:
const sortedIndex = (arr, n) => {
const isDescending = arr[0] > arr[arr.length - 1];
const index = arr.findIndex(el => (isDescending ? n >= el : n <= el));
return index === -1 ? arr.length : index;
};
console.log(sortedIndex([5, 3, 2, 1], 4));
console.log(sortedIndex([30, 50], 40));
Sample Output:
1 1
Flowchart:
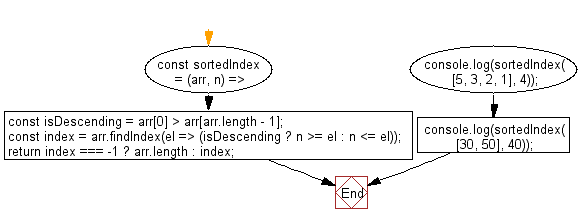
Live Demo:
See the Pen javascript-common-editor by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that finds the lowest index for inserting a value in a sorted array using a linear search.
- Write a JavaScript function that handles empty arrays by returning 0 as the insertion index.
- Write a JavaScript function that compares the element at each index with the target to determine the correct insertion point.
- Write a JavaScript function that simulates the insertion and returns the updated array order.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to Finds the index of a given element in a sorted array using the binary search algorithm.
Next: Write a JavaScript program to find the highest index at which a value should be inserted into an array in order to maintain its sort order.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.