JavaScript Exercises: Rotate the stack elements to the left
JavaScript Stack: Exercise-11 with Solution
Rotate Stack Left
Write a JavaScript program to rotate the stack elements to the left direction.
Sample Solution:
JavaScript Code:
class Stack {
constructor() {
this.items = [];
}
push(element) {
this.items.push(element);
}
pop() {
if (this.isEmpty())
return "Underflow";
return this.items.pop();
}
peek() {
if (this.isEmpty())
return "No elements in Stack";
return this.items[this.items.length - 1];
}
isEmpty() {
return this.items.length === 0;
}
rotateLeft() {
if (this.isEmpty()) {
return "Underflow";
}
let firstElement = this.items.shift();
this.items.push(firstElement);
return this.items;
}
displayStack(stack) {
console.log("Stack elements are:");
let str = "";
for (let i = 0; i < stack.items.length; i++)
str += stack.items[i] + " ";
return str.trim();
}
}
console.log("Initialize a stack:")
let stack = new Stack();
console.log("Input some elements on the stack:")
stack.push(1);
stack.push(2);
stack.push(3);
stack.push(4);
stack.push(5);
console.log(stack.displayStack(stack));
console.log("Rotate the stack elements to the left:")
console.log(stack.rotateLeft());
console.log("Again rotate the stack elements to the left:")
console.log(stack.rotateLeft());
console.log("Again rotate the stack elements to the left:")
console.log(stack.rotateLeft());
Sample Output:
Initialize a stack: Input some elements on the stack: Stack elements are: 1 2 3 4 5 Rotate the stack elements to the left: [2,3,4,5,1] Again rotate the stack elements to the left: [3,4,5,1,2] Again rotate the stack elements to the left: [4,5,1,2,3]
Flowchart:
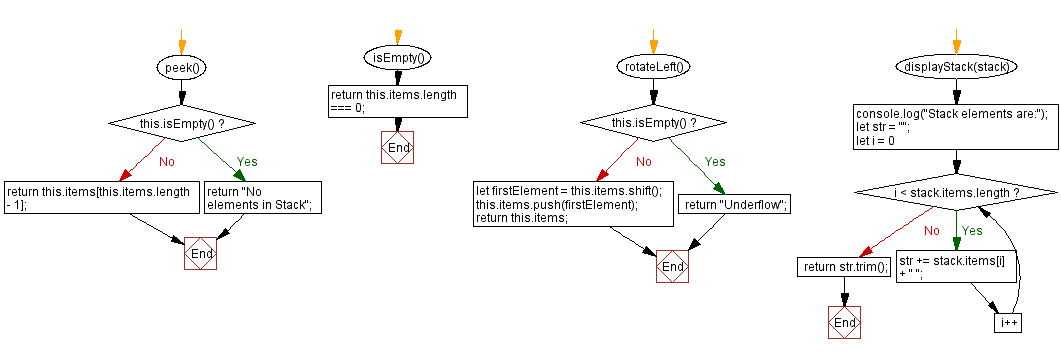
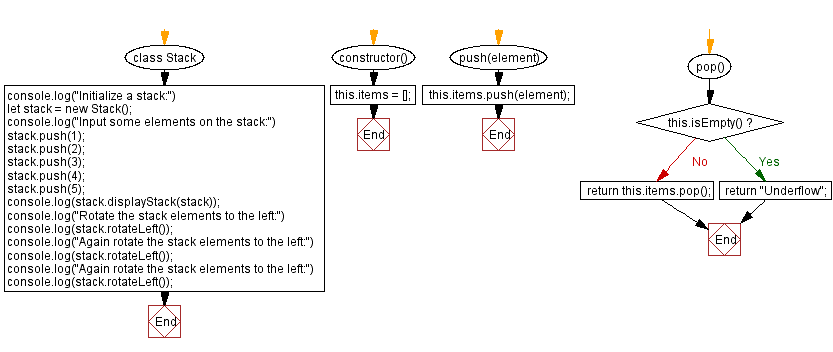
Live Demo:
* To run the code mouse over on Result panel and click on 'RERUN' button.*
Live Demo:
See the Pen javascript-stack-exercise-11 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that rotates the elements of a stack to the left by one position using shift and push operations.
- Write a JavaScript function that rotates a stack left by n positions and returns the modified stack.
- Write a JavaScript function that uses recursion to rotate a stack to the left without using extra space.
- Write a JavaScript function that verifies the left rotation by comparing the new order with the expected cyclic permutation.
Improve this sample solution and post your code through Disqus
Stack Previous: Top and bottom elements of a stack.
Stack Exercises Next: Rotate the stack elements to the right.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.