PHP Exercises: Check if a given array of integers contains no 3 or a 5
115. Array Contains No 3 or 5 Check
Write a PHP program to check if a given array of integers contains no 3 or a 5.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that takes an array of numbers as a parameter
function test($nums)
{
// Initialize variables $three and $five with values of false
$three = false;
$five = false;
// Iterate through the elements of the array using a for loop
for ($i = 0; $i < sizeof($nums); $i++)
{
// Check if the current element is equal to 3, set $three to true if true
if ($nums[$i] == 3) {$three = true;}
// Check if the current element is equal to 5, set $five to true if true
if ($nums[$i] == 5) {$five = true;}
// Check if both $three and $five are true, return false if true
if ($three && $five) return false;
}
// Return true if there are no consecutive occurrences of both 3 and 5, otherwise return false
return true;
}
// Use 'var_dump' to print the result of calling 'test' with different arrays
var_dump(test([5, 5, 5, 5, 5]));
var_dump(test([3, 3, 3, 3]));
var_dump(test([3, 3, 3, 5, 5, 5]));
var_dump(test([1, 6, 8, 10]));
?>
Sample Output:
bool(true) bool(true) bool(false) bool(true)
Flowchart:
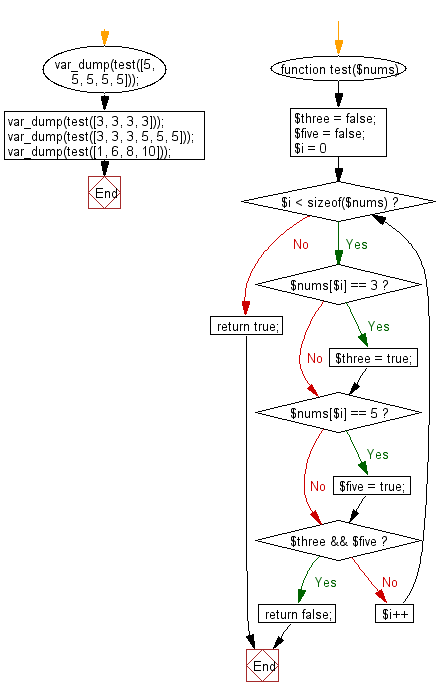
For more Practice: Solve these Related Problems:
- Write a PHP script to return true if an array does not contain any occurrences of 3 or 5.
- Write a PHP function to iterate over an array and output true only if neither 3 nor 5 is present.
- Write a PHP program to use logical conditions to verify that an array lacks both 3 and 5.
- Write a PHP script to employ array filtering to confirm an array is free of the values 3 and 5, then return a boolean.
Go to:
PREV : Contains 3 or 5 in Array Check.
NEXT : Contains Adjacent 3's or 5's Check.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.