PHP Exercises: Create new array from a given array of integers shifting all even numbers before all odd numbers
129. Shift Even Numbers Before Odds in Array
Write a PHP program to create new array from a given array of integers shifting all even numbers before all odd numbers.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that takes an array of numbers as a parameter
function test($numbers)
{
// Initialize a variable '$index' to keep track of the position of even numbers
$index = 0;
// Iterate through the elements of the input array using a for loop
for ($i = 0; $i < sizeof($numbers); $i++)
{
// Check if the current element is an even number
if ($numbers[$i] % 2 == 0)
{
// Swap the current even number with the one at the position indicated by '$index'
$temp = $numbers[$index];
$numbers[$index] = $numbers[$i];
$numbers[$i] = $temp;
// Increment '$index' to the next position for the next even number
$index++;
}
}
// Return the modified array with even numbers moved to the front
return $numbers;
}
// Call the 'test' function with an example array and store the result in the variable 'result'
$result = test([1, 2, 5, 3, 5, 4, 6, 9, 11]);
// Print the result array as a string
echo "New array: " . implode(',', $result);
?>
Sample Output:
New array: 2,4,6,3,5,1,5,9,11
Flowchart:
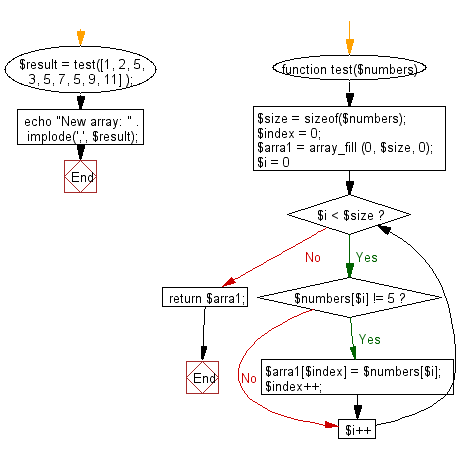
For more Practice: Solve these Related Problems:
- Write a PHP script to rearrange an array so that all even numbers come before all odd numbers while preserving internal order.
- Write a PHP function to partition an array into even and odd elements and then concatenate the two partitions.
- Write a PHP program to implement a stable partition algorithm that moves even values to the front of the array.
- Write a PHP script to iterate through an array and rebuild it with evens first followed by odds using loops.
Go to:
PREV : Replace 5 with 0 and Shift Zeros to Right.
NEXT : Check Non-Decreasing Order of Array.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.