PHP Exercises: Create a new array of given length using the odd numbers from a given array of positive integers
PHP Basic Algorithm: Exercise-136 with Solution
Write a PHP program to create a new array of given length using the odd numbers from a given array of positive integers.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that takes an array of numbers 'nums' and an integer 'count'
function test($nums, $count)
{
// Initialize an array 'evens' to store odd numbers, with size 'count'
$evens = [$count];
// Initialize a variable 'j' to track the index in the 'evens' array
$j = 0;
// Use a for loop to iterate through the elements of 'nums'
for ($i = 0; $j < $count; $i++)
{
// Check if the current number is odd
if ($nums[$i] % 2 != 0)
{
// If true, store the odd number in the 'evens' array and increment 'j'
$evens[$j] = $nums[$i];
$j++;
}
}
// Return the resulting 'evens' array
return $evens;
}
// Call the 'test' function with an example input and display the result
$result = test([1,2,3,5,7,9,10], 3);
echo "New array: " . implode(",", $result);
?>
Sample Output:
New array: 1,3,5
Flowchart:
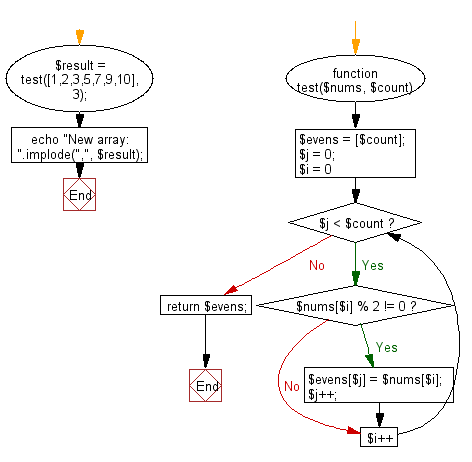
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to check a positive integer and return true if it contains a number 2.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics