PHP Exercises: Create a new string which is n copies of a given string
PHP Basic Algorithm: Exercise-25 with Solution
Write a PHP program to create a new string which is n (non-negative integer) copies of a given string.
Sample Solution:
PHP Code :
<?php
// Define a function that repeats a string $n times
function test($s, $n)
{
// Initialize an empty string to store the result
$result = "";
// Iterate $n times and concatenate the input string to the result
for ($i = 0; $i < $n; $i++) {
$result = $result . $s;
}
// Return the final result
return $result;
}
// Test the function with different inputs
echo test("JS", 2) . "\n";
echo test("JS", 3) . "\n";
echo test("JS", 1) . "\n";
?>
Explanation:
- Function Definition:
- The test function takes two parameters: $s (a string to repeat) and $n (the number of times to repeat it).
- Initialize Result Variable:
- An empty string "$result" is initialized to accumulate the repeated string.
- Loop to Repeat the String:
- A for loop runs $n times, concatenating $s to $result in each iteration. By the end of the loop, $result contains $s repeated $n times.
- Return Result:
- The function returns the final accumulated string stored in $result.
Output:
JSJS JSJSJS JS
Visual Presentation:
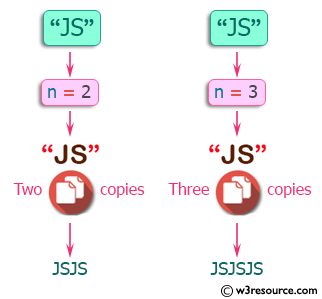
Flowchart:
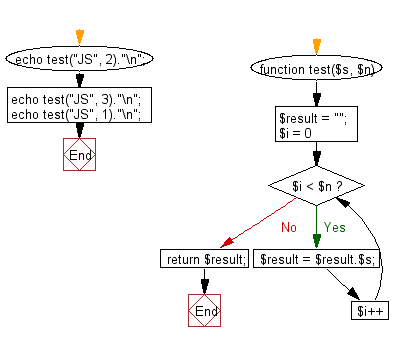
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to convert the last 3 characters of a given string in upper case. If the length of the string has less than 3 then uppercase all the characters.
Next: Write a PHP program to create a new string which is n (non-negative integer) copies of the the first 3 characters of a given string. If the length of the given string is less than 3 then return n copies of the string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/php-exercises/basic-algorithm/php-basic-algorithm-exercise-25.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics