PHP Exercises: Count the string "aa" in a given string and assume "aaa" contains two "aa"
PHP Basic Algorithm: Exercise-27 with Solution
Write a PHP program to count the string "aa" in a given string and assume "aaa" contains two "aa".
Sample Solution:
PHP Code :
<?php
// Define a function that counts the occurrences of the substring "aa" in the input string
function test($s)
{
// Initialize a counter for occurrences of "aa"
$ctr_aa = 0;
// Iterate through the string, checking for occurrences of "aa"
for ($i = 0; $i < (strlen($s) - 1); $i++) {
if (substr($s, $i, 2) == "aa") {
$ctr_aa++;
}
}
// Return the count of occurrences
return $ctr_aa;
}
// Test the function with different input strings
echo test("bbaaccaag") . "\n";
echo test("jjkiaaasew") . "\n";
echo test("JSaaakoiaa") . "\n";
?>
Explanation:
- Function Definition:
- The test function takes one parameter, $s, which is a string to check for occurrences of the substring "aa".
- Initialize Counter:
- A variable $ctr_aa is initialized to 0 to keep track of the number of times "aa" appears in the string.
- Loop Through String:
- A for loop iterates through the string from the start to the second-to-last character. The loop runs until strlen($s) - 1 to avoid an out-of-bounds error.
- Check for Substring "aa":
- Inside the loop, substr($s, $i, 2) checks each consecutive pair of characters. If the current two characters are "aa", the counter $ctr_aa is incremented by 1.
- Return Count:
- After the loop completes, the function returns the total count of "aa" occurrences.
Output:
2 2 3
Visual Presentation:
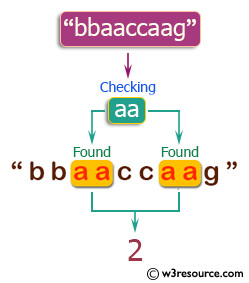
Flowchart:
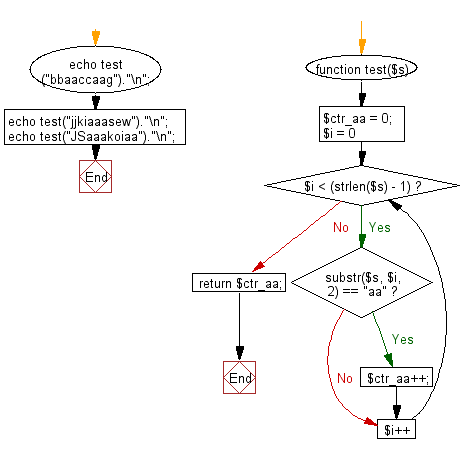
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to create a new string which is n (non-negative integer) copies of the the first 3 characters of a given string. If the length of the given string is less than 3 then return n copies of the string.
Next: Write a PHP program to check if the first appearance of "a" in a given string is immediately followed by another "a".
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/php-exercises/basic-algorithm/php-basic-algorithm-exercise-27.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics