PHP Exercises: Check whether the sequence of numbers 1, 2, 3 appears in a given array of integers somewhere
34. Check for Sequence 1,2,3 in Array
Write a PHP program to check whether the sequence of numbers 1, 2, 3 appears in a given array of integers somewhere.
Sample Solution:
PHP Code :
<?php
// Define a function that checks if the sequence 1, 2, 3 appears in an array
function test($nums)
{
// Iterate through the array to find the sequence
for ($i = 0; $i < sizeof($nums)-2; $i++)
{
// Check if the current, next, and next-next elements form the sequence 1, 2, 3
if ($nums[$i] == 1 && $nums[$i + 1] == 2 && $nums[$i + 2] == 3)
return true;
}
// If the sequence is not found, return false
return false;
}
// Test the function with different arrays
var_dump(test(array(1,1,2,3,1)));
var_dump(test(array(1,1,2,4,1)));
var_dump(test(array(1,1,2,1,2,3)));
?>
Explanation:
- Function Definition:
- The test function takes an array of numbers $nums as input and checks if the sequence [1, 2, 3] appears anywhere in the array.
- Loop through Array:
- The for loop iterates from the start of the array up to the third-to-last element to ensure there are enough elements left to check for the sequence.
- sizeof($nums) - 2 ensures we don't go out of bounds when checking three consecutive elements.
- Check for Sequence:
- Inside the loop, the code checks if three consecutive elements form the sequence [1, 2, 3] by comparing:
- $nums[$i] == 1
- $nums[$i + 1] == 2
- $nums[$i + 2] == 3
- If the sequence is found, the function returns true.
- Return False if Sequence Not Found:
- If the loop completes without finding the sequence [1, 2, 3], the function returns false.
Output:
bool(true) bool(false) bool(true)
Visual Presentation:
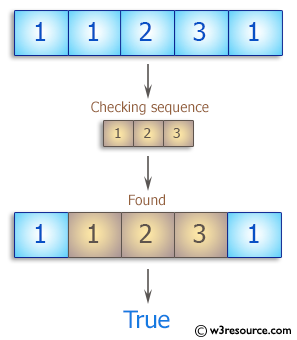
Flowchart:
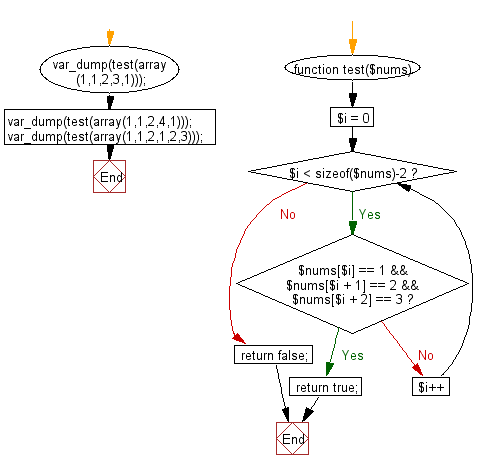
For more Practice: Solve these Related Problems:
- Write a PHP script to check if the sequence 1, 2, 3 appears consecutively anywhere in an integer array.
- Write a PHP function to scan an array for a specific sub-sequence and return a boolean result if found.
- Write a PHP program to search for contiguous numbers matching the pattern 1, 2, 3 using a sliding window technique.
- Write a PHP script to implement subarray matching logic to detect if the integer sequence 1,2,3 occurs in order.
Go to:
PREV : First Four Elements Array Element Match.
NEXT : Compare Strings for Matching 2-Character Substrings.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.