PHP Exercises: Create a new string of the characters at indexes 0,1,4,5,8,9 ... from a given string
36. New String from Specific Indexes
Write a PHP program to create a new string of the characters at indexes 0,1,4,5,8,9 ... from a given string.
Sample Solution:
PHP Code :
<?php
// Define a function that extracts substrings from a given string
function test($s)
{
// Initialize an empty string to store the result
$result = "";
// Iterate through the characters of the input string with a step size of 4
for ($i = 0; $i < strlen($s); $i = $i + 4)
{
// Calculate the end index of the substring
$c = $i + 2;
// Initialize a variable to store the substring length
$n = 0;
// Determine if the calculated end index is beyond the length of the string
$z = $c > strlen($s) ? 1 : 2;
// Update the substring length based on the condition
$n = $n + $z;
// Append the extracted substring to the result
$result = $result . substr($s, $i, $n);
}
// Return the final result
return $result;
}
// Test the function with different input strings
echo (test("Python"))."\n";
echo (test("JavaScript"))."\n";
echo (test("HTML"))."\n";
?>
Explanation:
- Function Definition:
- The test function takes a single string $s as input and extracts specific substrings based on certain conditions.
- Initialize Result String:
- An empty string $result is initialized to store the final result.
- Iterate with Step Size of 4:
- A for loop iterates through $s with a step size of 4, so i increments by 4 in each loop, meaning it processes every fourth character in the string.
- Calculate Substring End Index:
- For each iteration, c is set to i + 2, marking the end of a two-character substring starting at index i.
- Determine Substring Length:
- A variable $z determines the substring length:
- If c is beyond the end of the string, $z is set to 1.
- Otherwise, $z is set to 2, allowing the substring to be two characters long.
- $n is updated with this value of $z, making $n the actual length of the substring to extract.
- Extract and Append Substring:
- The substr($s, $i, $n) function extracts a substring starting at index i with length $n, and appends it to $result.
- Return the Result:
- After the loop completes, $result contains the concatenated substrings extracted from $s, which the function returns.
Output:
Pyon JaScpt HT
Visual Presentation:
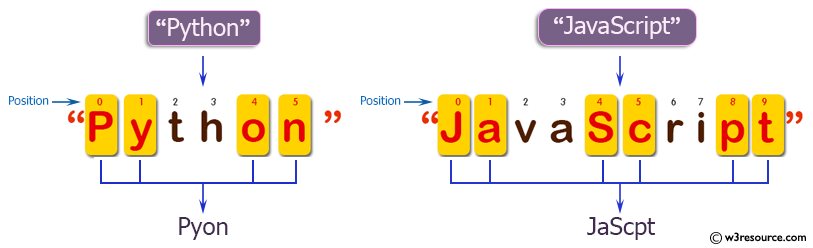
Flowchart:
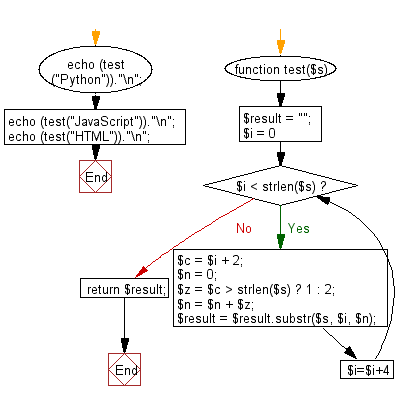
For more Practice: Solve these Related Problems:
- Write a PHP script to construct a new string using characters from specific indexes (0,1,4,5,8,9, etc.) of the input string.
- Write a PHP function to extract characters from an array of specified positions and concatenate them into a result.
- Write a PHP program to use a mapping of indexes to rebuild a string containing only selected characters.
- Write a PHP script to check the length and then assemble a new string based on fixed index positions from the original.
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to compare two given strings and return the number of the positions where they contain the same length 2 substring.
Next: Write a PHP program to count the number of two 5's are next to each other in an array of integers. Also count the situation where the second 5 is actually a 6.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.