PHP Exercises: Check a given integer and return true if it is within 10 of 100 or 200
PHP Basic Algorithm: Exercise-4 with Solution
Write a PHP program to check a given integer and return true if it is within 10 of 100 or 200.
Sample Solution:
PHP Code :
<?php
// Define a function named "test" that takes a parameter $x
function test($x)
{
// Use the absolute value function (abs) to check if the absolute difference between $x and 100 is less than or equal to 10
// OR if the absolute difference between $x and 200 is less than or equal to 10
if (abs($x - 100) <= 10 || abs($x - 200) <= 10)
// If true, return true
return true;
// If false, return false
return false;
}
// Use var_dump to print the result of calling test with argument 103
var_dump(test(103));
// Use var_dump to print the result of calling test with argument 90
var_dump(test(90));
// Use var_dump to print the result of calling test with argument 89
var_dump(test(89));
?>
Sample Output:
bool(true) bool(true) bool(false)
Flowchart:
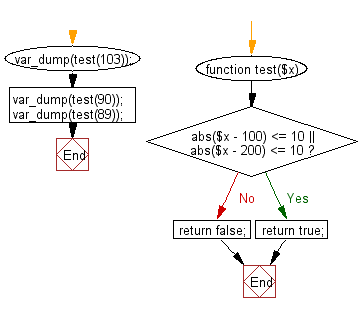
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to check two given integers, and return true if one of them is 30 or if their sum is 30.
Next: Write a PHP program to create a new string where 'if' is added to the front of a given string. If the string already begins with 'if', return the string unchanged.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics