PHP Exercises: Accept two integers and return true if either one is 5 or their sum or difference is 5
PHP Basic Algorithm: Exercise-40 with Solution
Write a PHP program that accept two integers and return true if either one is 5 or their sum or difference is 5.
Sample Solution:
PHP Code :
<?php
// Define a function that checks conditions related to the numbers
function test($x, $y)
{
// Check if $x is 5 or $y is 5 or the sum of $x and $y is 5
// or the absolute difference between $x and $y is 5
return $x == 5 || $y == 5 || $x + $y == 5 || abs($x - $y) == 5;
}
// Test the function with different pairs of numbers
var_dump(test(5, 4));
var_dump(test(4, 3));
var_dump(test(1, 4));
?>
Explanation:
- Function Definition:
- The test function takes two numbers, $x and $y, as parameters and checks several conditions.
- Conditions Checked:
- It checks if:
- $x is equal to 5.
- $y is equal to 5.
- The sum of $x and $y is 5.
- The absolute difference between $x and $y is 5.
- If any of these conditions are true, the function returns true; otherwise, it returns false.
Output:
bool(true) bool(false) bool(true)
Visual Presentation:
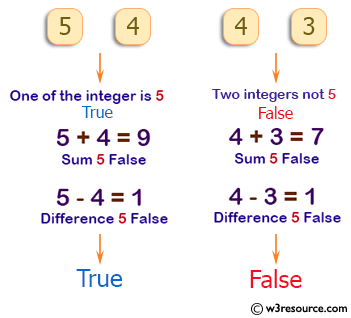
Flowchart:
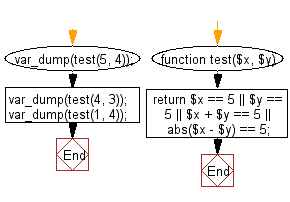
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to compute the sum of the two given integers. If the sum is in the range 10..20 inclusive return 30.
Next: Write a PHP program to test if a given non-negative number is a multiple of 13 or it is one more than a multiple of 13.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/php-exercises/basic-algorithm/php-basic-algorithm-exercise-40.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics