PHP Exercises: Check whether a given string starts with "F" or ends with "B"
45. FizzBuzz String Start with F and/or End with B
Write a PHP program to check whether a given string starts with "F" or ends with "B". If the string starts with "F" return "Fizz" and return "Buzz" if it ends with "B" If the string starts with "F" and ends with "B" return "FizzBuzz". In other cases return the original string.
Sample Solution:
PHP Code :
<?php
// Define a function that checks the given string for specific conditions and returns corresponding values
function test($s)
{
// Check if the first character is "F" AND the last character is "B"
// If true, return "FizzBuzz"
if ((substr($s, 0, 1) == "F") && (substr($s, strlen($s) - 1, 1) == "B")) {
return "FizzBuzz";
}
// Check if the first character is "F"
// If true, return "Fizz"
else if (substr($s, 0, 1) == "F") {
return "Fizz";
}
// Check if the last character is "B"
// If true, return "Buzz"
else if (substr($s, strlen($s) - 1, 1) == "B") {
return "Buzz";
}
// If none of the conditions are met, return the original string
else {
return $s;
}
}
// Test the function with different strings
echo test("FizzBuzz")."\n";
echo test("Fizz")."\n";
echo test("Buzz")."\n";
echo test("Founder")."\n";
?>
Explanation:
- Function Definition:
- The test function checks the input string $s to see if it meets certain conditions based on its first and last characters.
- Conditions Checked:
- First Condition: If the first character of $s is "F" and the last character is "B", it returns "FizzBuzz".
- Second Condition: If only the first character is "F", it returns "Fizz".
- Third Condition: If only the last character is "B", it returns "Buzz".
- Else: If none of the conditions are met, it returns the original string $s.
Output:
Fizz Fizz Buzz Fizz
Visual Presentation:
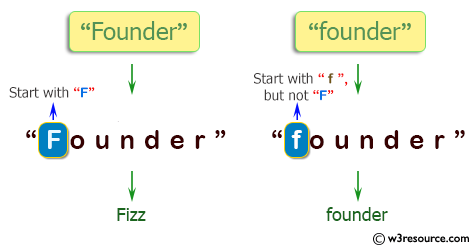
Flowchart:
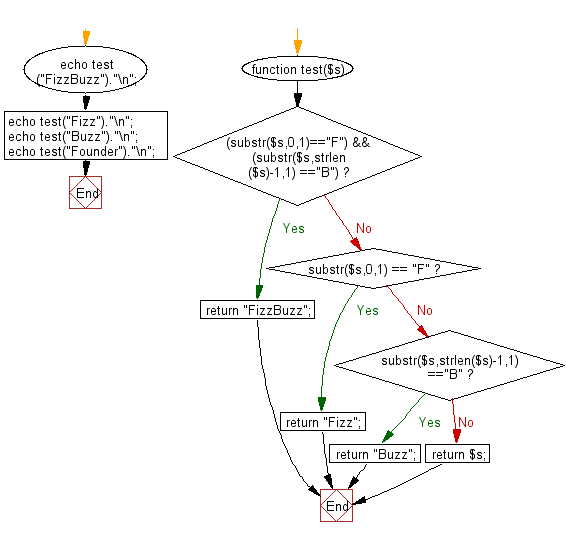
For more Practice: Solve these Related Problems:
- Write a PHP script to evaluate a string and return "Fizz" if it starts with "F", "Buzz" if it ends with "B", and "FizzBuzz" if both conditions are met.
- Write a PHP function to check the first and last characters of an input string and map those to special output strings per FizzBuzz rules.
- Write a PHP program to process a string and conditionally return custom values based on its prefix and suffix without using built-in functions.
- Write a PHP script to implement multiple condition checks on a string to output "Fizz", "Buzz", or "FizzBuzz", ensuring the correct order of evaluation.
Go to:
PREV : Sum with Special Range Override (10..20?18).
NEXT : Two Integers Sum to Third Check.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.