PHP Exercises: Compute the sum of two given non-negative integers x and y as long as the sum has the same number of digits as x
53. Sum with Digit-Length Constraint
Write a PHP program to compute the sum of two given non-negative integers x and y as long as the sum has the same number of digits as x. If the sum has more digits than x then return x without y.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that compares the length of the sum of two numbers with the length of the first number
// If the sum has a greater length, return the first number; otherwise, return the sum
function test($x, $y)
{
// Check if the length of the sum of $x and $y is greater than the length of $x
// If true, return $x; otherwise, return the sum of $x and $y
return strlen((string)($x + $y)) > strlen((string)$x) ? $x : $x + $y;
}
// Test the 'test' function with different input values and display the results
echo (test(4, 5))."\n";
echo (test(7, 4))."\n";
echo (test(10, 10))."\n";
?>
Explanation:
- Function Definition:
- The test function takes two arguments, $x and $y, and checks the length of the sum of these two numbers compared to the length of $x.
- Condition:
- The function converts both the sum of $x and $y and $x individually to strings and compares their lengths.
- If the length of the sum is greater than the length of $x, it returns $x.
- Otherwise, it returns the sum of $x and $y.
Output:
9 7 20
Flowchart:
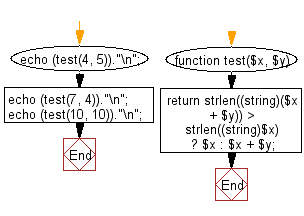
For more Practice: Solve these Related Problems:
- Write a PHP script to calculate the sum of two non-negative integers and return the original first integer if the digit count of the sum exceeds that of the first input.
- Write a PHP function to add two numbers and compare the digit length of their sum with the first number, outputting the first number if exceeded.
- Write a PHP program to validate two inputs by summing them and then conditionally return either their sum or the first integer based on digit length.
- Write a PHP script to compute the sum, convert numbers to strings, and compare lengths to decide whether to include the second number in the output.
Go to:
PREV : Shared Digit in Two Integers.
NEXT : Sum of Three with Duplicate Override.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.