PHP Exercises: Create a new string of length 2 starting at the given index of a given string
73. Substring of Length 2 at Given Index
Write a PHP program to create a new string of length 2 starting at the given index of a given string.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that extracts a substring of length 2 starting from the specified index in the input string
// If the index + 2 exceeds the length of the string, extract the first 2 characters
function test($s1, $index)
{
// Use substr to extract a substring of length 2 starting from the specified index
// If the index + 2 exceeds the length of the string, extract the first 2 characters
return $index + 2 <= strlen($s1) ? substr($s1, $index, 2) : substr($s1, 0, 2);
}
// Test the 'test' function with different strings and index values, then display the results using echo
echo test("Hello", 1)."\n";
echo test("Python", 2)."\n";
echo test("on", 1)."\n";
?>
Explanation:
- Function Definition:
- A function named test is defined with two parameters:
- $s1: the input string.
- $index: the starting position for extracting a substring.
- Substring Extraction:
- The function uses a conditional expression to decide which substring to extract:
- If $index + 2 is less than or equal to the length of $s1, substr($s1, $index, 2) extracts two characters from the specified index.
- If $index + 2 exceeds the length of $s1, substr($s1, 0, 2) extracts the first two characters of the string.
Output:
el th on
Flowchart:
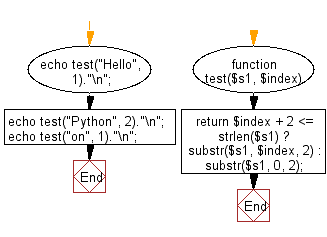
For more Practice: Solve these Related Problems:
- Write a PHP script to extract a substring of length 2 starting at a specified index from a given string.
- Write a PHP function to validate the given index and return exactly two characters from that position.
- Write a PHP program to use substr to pick a 2-letter slice from a string at the user-defined index.
- Write a PHP script to implement error handling while extracting a two-character segment from a provided index.
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to create a new string using the first and last n characters from a given string of length at least n.
Next: Write a PHP program to create a new string taking 3 characters from the middle of a given string at least 3.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.