PHP Challenges: Check whether a given positive integer is a power of four
PHP Challenges - 1: Exercise-3 with Solution
Write a PHP program to check whether a given positive integer is a power of four.
Input : 4
Explanation :
Sample Solution :
PHP Code :
<?php
// Function to check if a number is a power of four
function is_Power_of_four($n)
{
// Assign $n to a variable $x for manipulation
$x = $n;
// Loop until $x is divisible by 4
while ($x % 4 == 0) {
// Divide $x by 4
$x /= 4;
}
// Check if $x is equal to 1 after the loop
if ($x == 1) {
// If true, $n is a power of 4
return "$n is power of 4";
} else {
// If false, $n is not a power of 4
return "$n is not power of 4";
}
}
// Testing the function with different inputs
print_r(is_Power_of_four(4)."\n");
print_r(is_Power_of_four(36)."\n");
print_r(is_Power_of_four(16)."\n");
?>
Explanation:
Here is a brief explanation of the above PHP code:
- Define a function named 'is_Power_of_four' that takes a single parameter '$n', which is the number to be checked for being a power of four.
- Inside the function:
- It assigns the value of '$n' to a variable '$x'.
- It enters a while loop that continues as long as '$x' is divisible by 4. Inside the loop, it divides '$x' by 4 in each iteration.
- After the loop, it checks if '$x' is equal to 1. If it is, then '$n' is a power of 4, so it returns a string indicating that. Otherwise, it returns a string indicating that '$n' is not a power of 4.
- Finally, the function is tested with three different values (4, 36, and 16) using the 'print_r' function to display the results of each test.
Sample Output:
4 is power of 4 36 is not power of 4 16 is power of 4
Flowchart:
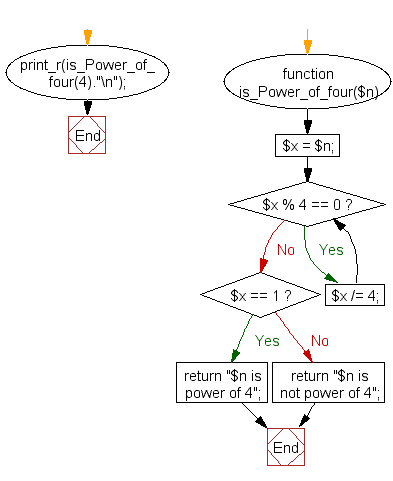
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to check whether a given positive integer is a power of three.
Next: Write a PHP program to check whether an integer is the power of another integer.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/php-exercises/challenges/1/php-challenges-1-exercise-3.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics