PHP Challenges: Find three numbers from an array such that the sum of three consecutive numbers equal to zero
Write a PHP program to find three numbers from an array such that the sum of three consecutive numbers equal to zero.
Input : (-1,0,1,2,-1,-4)
Explanation :
Sample Solution :
PHP Code :
<?php
// Function to find triplets whose sum equals zero
function three_Sum_zero($arr)
{
// Calculate the number of elements in the array minus 2
$count = count($arr) - 2;
// Initialize an empty array to store the result
$result=[];
// Iterate through the array
for ($x = 0; $x < $count; $x++)
{
// Check if the sum of the current element and the next two elements equals zero
if ($arr[$x] + $arr[$x+1] + $arr[$x+2] == 0)
{
// If true, push the triplet to the result array
array_push($result, "{$arr[$x]} + {$arr[$x+1]} + {$arr[$x+2]} = 0");
}
}
// Return the array of triplets whose sum equals zero
return $result;
}
// Define two arrays of numbers
$nums1= array(-1,0,1,2,-1,-4);
$nums2 = array(-25,-10,-7,-3,2,4,8,10);
// Test the three_Sum_zero function with the defined arrays and print the result
print_r(three_Sum_zero($nums1));
print_r(three_Sum_zero($nums2));
?>
Explanation:
Here is a brief explanation of the above PHP code:
- The code defines a function named 'three_Sum_zero' which takes an array '$arr' as input.
- Inside the function:
- It calculates the number of elements in the array minus 2 and stores it in '$count'
- It initializes an empty array '$result' to store the triplets whose sum equals zero.
- It iterates through the array using a 'for' loop.
- For each iteration, it checks if the sum of the current element and the next two elements equals zero.
- If the condition is true, it formats the triplet as a string and pushes it into the '$result' array.
- Finally, it returns the '$result' array containing all the triplets whose sum equals zero.
- Two arrays '$nums1' and '$nums2' are defined with different sets of numbers.
- The 'three_Sum_zero' function is called twice with these arrays as input.
- The results of the function calls are printed using 'print_r()', which displays the array of triplets whose sum equals zero for each input array.
Sample Output:
Array ( [0] => -1 + 0 + 1 = 0 ) Array ( )
Flowchart:
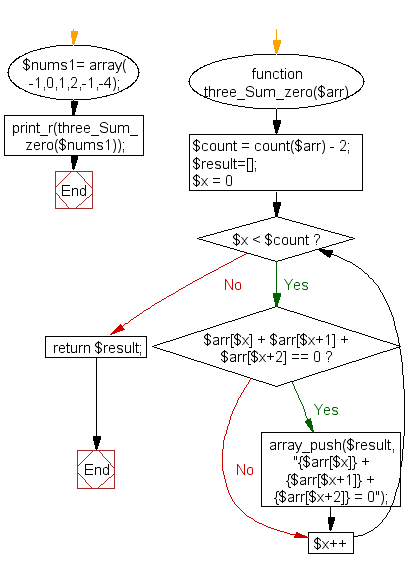
Go to:
PREV : Write a PHP program to find a missing number(s) from an array.
NEXT : Write a PHP program to find three numbers from an array such that the sum of three consecutive numbers equal to a given number.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.