PHP Challenges: Find a single number in a array that doesn't occur twice
Write a PHP program to find a single number in an array that doesn't occur twice.
Input : array(5, 3, 4, 3, 4)
Explanation :
Sample Solution :
PHP Code :
<?php
// Function to find the single number in an array
function single_number($arr)
{
// Initialize $result with the first element of the array
$result = $arr[0];
// Iterate through the array starting from the second element
for ($i = 1; $i < sizeof($arr); $i++)
{
// Use bitwise XOR operation to find the single number
$result = $result ^ $arr[$i];
}
// Return the single number found
return $result;
}
// Define two arrays
$arr1 = array(5, 3, 4, 3, 4);
$arr2 = array(3, 2, 5, 2, 1, 5, 3);
// Print the first array
print_r($arr1);
// Print the single number found in the first array
print_r('Single Number: ' . single_number($arr1) . "\n");
// Print the second array
print_r($arr2);
// Print the single number found in the second array
print_r('Single Number: ' . single_number($arr2) . "\n");
?>
Explanation:
Here is a brief explanation of the above PHP code:
- The code defines a function named "single_number()" which finds the single number in an array where every element appears twice except for one. It takes an array '$arr' as input.
- Inside the function:
- It initializes '$result' with the first element of the array.
- It iterates through the array starting with the second element.
- Within each iteration, it uses the bitwise XOR (^) operation to find the single number.
- After the loop, it returns the single number found.
- Function Call & Testing:
- Two arrays '$arr1' and '$arr2' are defined with different sets of numbers.
- The "single_number()" function is called twice with these arrays as input.
- The input arrays and single numbers are printed using "print_r()".
Sample Output:
Array ( [0] => 5 [1] => 3 [2] => 4 [3] => 3 [4] => 4 ) Single Number: 5 Array ( [0] => 3 [1] => 2 [2] => 5 [3] => 2 [4] => 1 [5] => 5 [6] => 3 ) Single Number: 1
Flowchart:
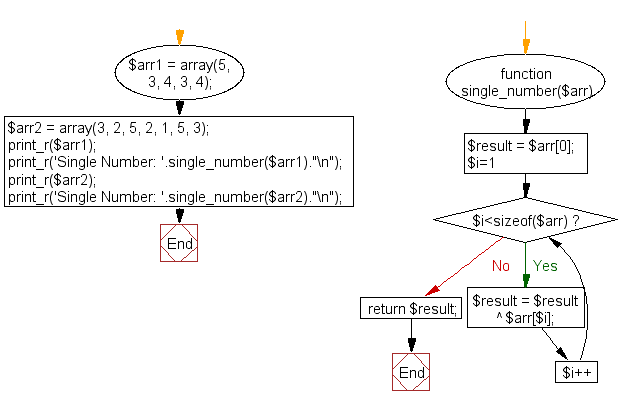
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to compute and return the square root of a given number.
Next: Write a PHP program to find a single element in an array where every element appears three times except for one.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.