PHP Array Exercises : Change the array values to upper or lower case
12. Change Array Values to Lower and Upper Case
Write a PHP function to change the following array's all values to upper or lower case.
Sample arrays :
$Color = array('A' => 'Blue', 'B' => 'Green', 'c' => 'Red');
Sample Solution:
PHP Code:
<?php
// Define a function named 'array_change_value_case' that changes the case of array values
function array_change_value_case($input, $ucase)
{
// Store the desired case (CASE_UPPER or CASE_LOWER) in the variable $case
$case = $ucase;
// Initialize an empty array to store the new values
$narray = array();
// Check if the input is not an array
if (!is_array($input))
{
// If not an array, return an empty array
return $narray;
}
// Iterate through the input array
foreach ($input as $key => $value)
{
// Check if the current value is an array
if (is_array($value))
{
// If it's an array, recursively call the function on the sub-array
$narray[$key] = array_change_value_case($value, $case);
// Continue to the next iteration
continue;
}
// Change the case of the current value based on the specified case
$narray[$key] = ($case == CASE_UPPER ? strtoupper($value) : strtolower($value));
}
// Return the new array with changed case values
return $narray;
}
// Define an associative array $Color with keys 'A', 'B', and 'c'
$Color = array('A' => 'Blue', 'B' => 'Green', 'c' => 'Red');
// Output a message indicating the start of the section for the actual array
echo 'Actual array ';
// Display the original array $Color
print_r($Color);
// Output a message indicating that values are in lower case
echo 'Values are in lower case.';
// Call the array_change_value_case function with CASE_LOWER and display the result
$myColor = array_change_value_case($Color, CASE_LOWER);
print_r($myColor);
// Output a message indicating that values are in upper case
echo 'Values are in upper case.';
// Call the array_change_value_case function with CASE_UPPER and display the result
$myColor = array_change_value_case($Color, CASE_UPPER);
print_r($myColor);
?>
Output:
Actual array Array ( [A] => Blue [B] => Green [c] => Red ) Values are in lower case.Array ( [A] => blue [B] => green [c] => red ) Values are in upper case.Array ( [A] => BLUE [B] => GREEN [c] => RED )
Flowchart:
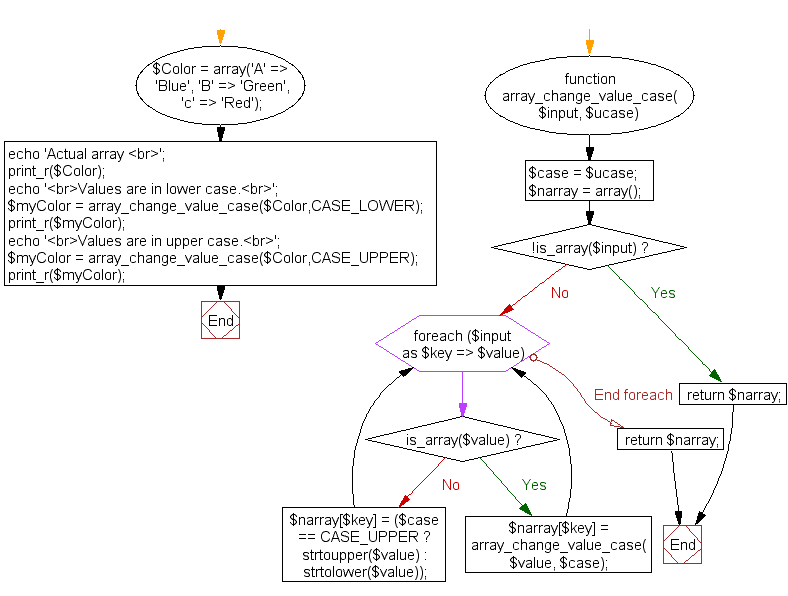
For more Practice: Solve these Related Problems:
- Write a PHP function to convert all values in an associative array to lowercase using array_map and display the result.
- Write a PHP script to iterate over an array and toggle each value between upper and lower case, then output both versions.
- Write a PHP program to compare the results of converting an array’s values to lowercase versus uppercase, then display both arrays side-by-side.
- Write a PHP script to implement a function that accepts an array and a desired case ("upper" or "lower") and returns the transformed array.
Go to:
PREV : Merge Arrays by Index.
NEXT : Display Numbers Divisible by 4 Without Control Statements.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.