PHP Array Exercises : Floor decimal numbers with precision
PHP Array: Exercise-18 with Solution
Write a PHP function to floor decimal numbers with precision.
Note: Accept three parameters number, precision, and $separator
Sample Data :
1.155, 2, "."
100.25781, 4, "."
-2.9636, 3, "."
Sample Solution:
PHP Code:
<?php
// Define a function named 'floorDec' that rounds down a decimal number to a specified precision using a given separator
function floorDec($number, $precision, $separator)
{
// Split the decimal number into integer and fractional parts using the specified separator
$number_part = explode($separator, $number);
// Replace a portion of the fractional part to achieve the desired precision
$number_part[1] = substr_replace($number_part[1], $separator, $precision, 0);
// If the integer part is non-negative, use 'floor' to round down the fractional part
if ($number_part[0] >= 0) {
$number_part[1] = floor($number_part[1]);
}
// If the integer part is negative, use 'ceil' to round up the fractional part
else {
$number_part[1] = ceil($number_part[1]);
}
// Combine the modified integer and fractional parts and return the result
$ceil_number = array($number_part[0], $number_part[1]);
return implode($separator, $ceil_number);
}
// Call the 'floorDec' function with examples and print the results
print_r(floorDec(1.155, 2, ".") . "\n");
print_r(floorDec(100.25781, 4, ".") . "\n");
print_r(floorDec(-2.9636, 3, ".") . "\n");
?>
Output:
1.15 100.2578 -2.964
Flowchart:
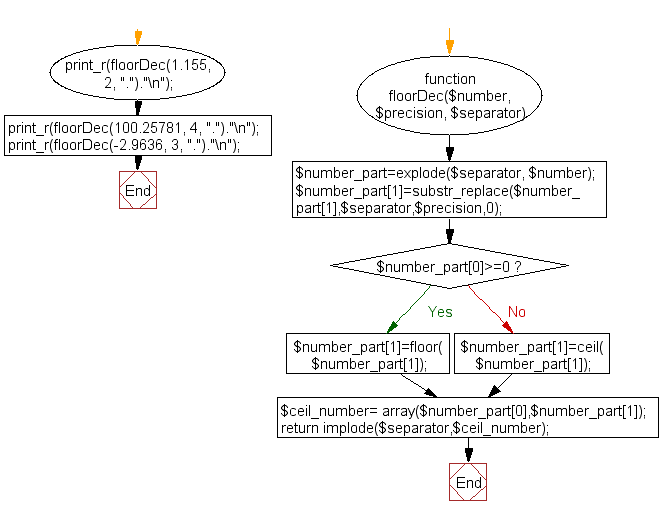
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP function that returns the lowest integer that is not 0.
Next: Write a PHP script to print "second" and Red from the specified array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/php-exercises/php-array-exercise-18.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics