PHP Array Exercises : Create a letter range with arbitrary length
30. Create Letter Range with Arbitrary Length
Write a PHP program to create a letter range with arbitrary length.
Sample Solution:
PHP Code:
<?php
// Define a function named letter_range that takes a length as input
function letter_range($length)
{
// Initialize an empty array to store the result
$data_range = array();
// Create an array of uppercase letters from 'A' to 'Z'
$letters = range('A', 'Z');
// Iterate through the specified length
for($i=0; $i<$length; $i++)
{
// Calculate the starting position for each iteration
$position = $i * 26;
// Iterate through the letters and generate combinations
foreach($letters as $ii => $letter)
{
// Increment the position
$position++;
// Check if the position is within the specified length
if($position <= $length)
// Add the generated combination to the result array
$data_range[] = ($position > 26 ? $data_range[$i-1] : '').$letter;
}
}
// Return the array containing the generated letter combinations
return $data_range;
}
// Test the function with a length of 7 and print the result
print_r(letter_range(7));
?>
Output:
Array ( [0] => A [1] => B [2] => C [3] => D [4] => E [5] => F [6] => G )
Flowchart:
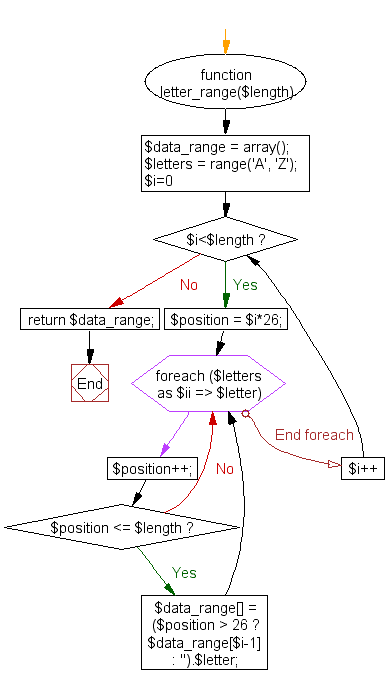
For more Practice: Solve these Related Problems:
- Write a PHP script to generate an array containing a range of letters between two given characters with a specified interval.
- Write a PHP function to create a sequential list of letters with an arbitrary length, supporting both uppercase and lowercase.
- Write a PHP program to dynamically generate a letter range and then output the concatenated result as a string.
- Write a PHP script to customize letter ranges using a starting letter, ending letter, and step value, then display the generated range.
Go to:
PREV : Array Range from String.
NEXT : Get Index of Highest Value in Associative Array.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.