PHP Array Exercises : Remove a specified duplicate entry from an array
Write a PHP a function to remove a specified duplicate entry from an array.
Sample Solution:
PHP Code:
<?php
// Define a function to remove a specified number of occurrences of a value from an array
function array_uniq($my_array, $value)
{
$count = 0;
// Iterate through the array
foreach($my_array as $array_key => $array_value)
{
// If more than 0 occurrences and the current value matches the specified value, unset it
if (($count > 0) && ($array_value == $value))
{
unset($my_array[$array_key]);
}
// If the current value matches the specified value, increment the count
if ($array_value == $value) $count++;
}
// Remove any null or empty elements from the array
return array_filter($my_array);
}
// Example usage: an array of numbers
$numbers = array(4, 5, 6, 7, 4, 7, 8);
// Call the function with the array and a value to remove
print_r(array_uniq($numbers, 7));
?>
Output:
Array ( [0] => 4 [1] => 5 [2] => 6 [3] => 7 [4] => 4 [6] => 8 )
Flowchart:
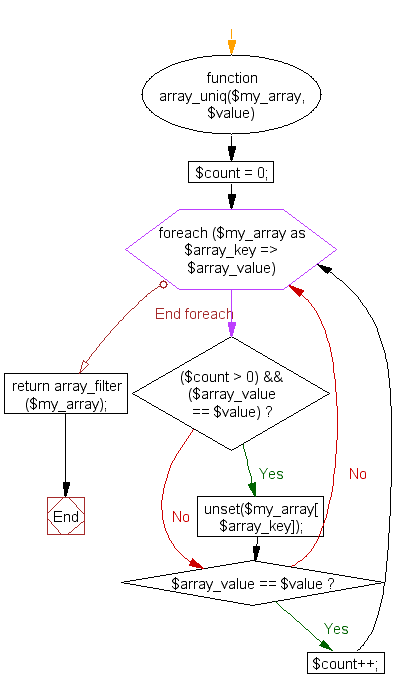
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP script to merge two commas separated lists with unique value only.
Next: Write a PHP script to do a multi-dimensional difference, i.e. returns values of the first array that are not in the second array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.