PHP Exercises: Capture a variable number of arguments to a given function
102. Capture a Variable Number of Arguments
Write a PHP program to capture a variable number of arguments to a given function.
Sample Solution:
PHP Code:
<?php
// Licence: https://bit.ly/2CFA5XY
// Function definition for 'variadicFunction' that takes an array of operands as a parameter
function variadicFunction($operands)
{
// Initialize a variable to store the sum of operands
$sum = 0;
// Iterate through each operand in the array and add it to the sum
foreach($operands as $singleOperand) {
$sum += $singleOperand;
}
// Return the final sum
return $sum;
}
// Call 'variadicFunction' with an array containing two operands and display the result using 'var_dump'
var_dump(variadicFunction([1, 2]));
// Call 'variadicFunction' with an array containing four operands and display the result using 'var_dump'
var_dump(variadicFunction([1, 2, 3, 4]));
?>
Explanation:
- Function Definition:
- The variadicFunction is defined to take a single parameter $operands, which is expected to be an array of numbers.
- Variable Initialization:
- A variable $sum is initialized to 0 to hold the cumulative sum of the operands.
- Iterate Over Operands:
- A foreach loop iterates through each element in the $operands array.
- Each element (referred to as $singleOperand) is added to the $sum.
- Return Sum:
- After the loop completes, the function returns the total sum of the operands.
- First Function Call:
- The function is called with an array containing two elements [1, 2].
- The result is displayed using var_dump, which outputs int(3).
- Second Function Call:
- The function is called again with an array containing four elements [1, 2, 3, 4].
- The result is displayed using var_dump, which outputs int(10).
Output:
int(3) int(10)
Flowchart:
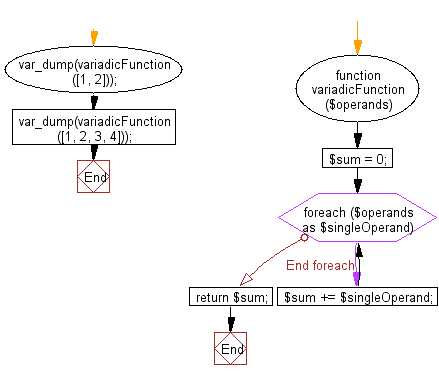
For more Practice: Solve these Related Problems:
- Write a PHP script to create a function that captures any number of arguments using func_get_args and processes them.
- Write a PHP function to sum an arbitrary number of numeric arguments and return the total.
- Write a PHP script to demonstrate variable argument handling by iterating over all passed parameters.
- Write a PHP function to capture, count, and display the arguments provided to it regardless of their number.
Go to:
PREV : Call a Function Only Once
NEXT : PHP Array Exercises Home.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.