PHP Exercises : Create a HTML form and accept the user name and display the name
4. Basic HTML Form and Echo Name
Create a simple HTML form and accept the user name and display the name through PHP echo statement.
HTML form: A webform or HTML form on a web page allows a user to enter data that is sent to a server for processing. Forms can resemble paper or database forms because web users fill out the forms using checkboxes, radio buttons, or text fields. For example, forms can be used to enter railway or credit card data to purchase a product, or can be used to retrieve search results from a search engine.
Sample Solution:
PHP Code:
<!DOCTYPE html>
<html>
<head>
<title></title>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
</head>
<body>
<!-- HTML form with POST method -->
<form method='POST'>
<h2>Please input your name:</h2>
<!-- Text input field for the name -->
<input type="text" name="name">
<!-- Submit button to submit the form -->
<input type="submit" value="Submit Name">
</form>
<?php
// Check if the form is submitted and 'name' is set in $_POST
if ($_SERVER["REQUEST_METHOD"] == "POST" && isset($_POST['name'])) {
// Retrieve name from the form and store it in a local variable
$name = $_POST['name'];
// Display a greeting message with the entered name
echo "<h3> Hello $name </h3>";
}
?>
</body>
</html>
Sample Output:
View the output in the browser
Explanation:
Here is a breakdown of the above PHP code:
- HTML Structure:
- The code starts with the usual HTML structure, including a head section with a title and character set information.
- Form Section:
- Inside the body, there is an HTML form with the 'method' attribute set to 'POST'. This form has an input field where users can enter their name and a submit button to submit the form.
- PHP Section:
- The PHP code begins with <?php and ends with ?>. It is embedded within the HTML and will be executed on the server side.
- The PHP code checks if the form has been submitted ($_SERVER["REQUEST_METHOD"] == "POST") and if the 'name' field is set in the $_POST array (isset($_POST['name'])).
- If the conditions are met, it retrieves the entered name from the '$_POST' array, stores it in a local variable '$name', and then displays a greeting message using 'echo'.
- The greeting message is wrapped in HTML tags (<h3>) and includes the entered name. This message will only be displayed if the form has been submitted and the 'name' field is not empty.
- End of HTML:
- The HTML body and document end tags (</body> and </html>) close the document.
Flowchart:
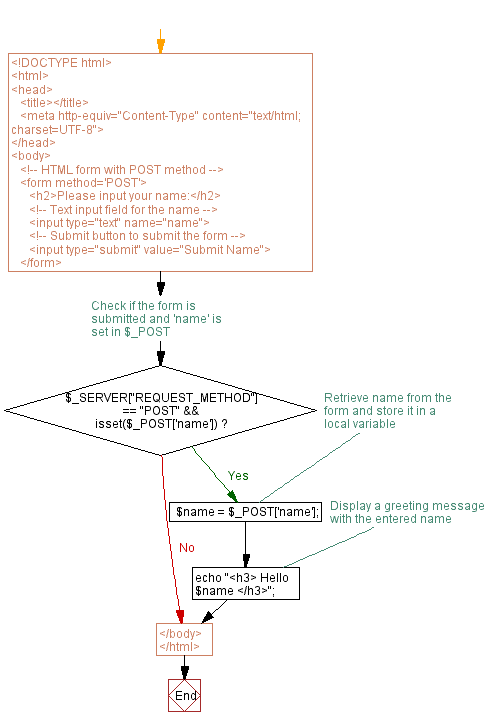
For more Practice: Solve these Related Problems:
- Write a PHP script to capture a user's name via an HTML form and display a greeting message with input validation.
- Write a PHP script to collect a user's full name from a form, then output the name in uppercase and reversed format.
- Write a PHP script to accept a name input and output a personalized welcome message after trimming and sanitizing the input.
- Write a PHP script to build a form that captures a first and last name and then echoes a formatted full name.
Go to:
PREV : Inject Variable in HTML Template.
NEXT : Get Client IP Address.
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.