PHP Date Exercises : Time difference in days and years, months, days, hours, minutes, seconds between two dates
11. Time Difference in Detailed Units
Write a PHP script to get time difference in days and years, months, days, hours, minutes, seconds between two dates.
Note : Use DateTime class.
Sample Solution:
PHP Code:
<?php
$date1 = new DateTime('2012-06-01 02:12:51'); // Creating a DateTime object for the first date.
$date2 = $date1->diff(new DateTime('2014-05-12 11:10:00')); // Calculating the difference between two dates.
echo $date2->days.'Total days'."\n"; // Outputting the total number of days between the two dates.
echo $date2->y.' years'."\n"; // Outputting the number of years in the difference.
echo $date2->m.' months'."\n"; // Outputting the number of months in the difference.
echo $date2->d.' days'."\n"; // Outputting the number of days in the difference.
echo $date2->h.' hours'."\n"; // Outputting the number of hours in the difference.
echo $date2->i.' minutes'."\n"; // Outputting the number of minutes in the difference.
echo $date2->s.' seconds'."\n"; // Outputting the number of seconds in the difference.
?>
Output:
710Total days 1 years 11 months 10 days 8 hours 57 minutes 9 seconds
Explanation:
In the exercise above,
- $date1 = new DateTime('2012-06-01 02:12:51');: Create a DateTime object representing June 1, 2012, at 02:12:51.
- $date2 = $date1->diff(new DateTime('2014-05-12 11:10:00'));: Calculates the difference between the DateTime object $date1 and the date May 12, 2014, at 11:10:00.
- $date2->days: Get the total number of days in the difference between the two dates.
- $date2->y, $date2->m, $date2->d, $date2->h, $date2->i, $date2->s: Get the number of years, months, days, hours, minutes, and seconds in the difference, respectively.
Flowchart :
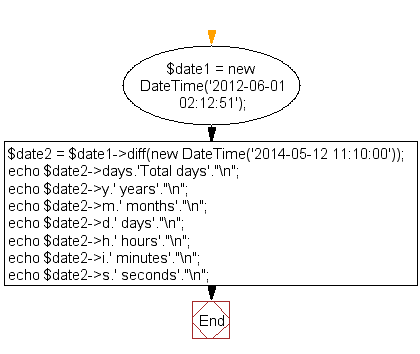
For more Practice: Solve these Related Problems:
- Write a PHP script to calculate the difference between two dates using DateTime objects and output the difference in years, months, days, hours, minutes, and seconds.
- Write a PHP function that accepts two date strings and returns an associative array with detailed time differences between them.
- Write a PHP program that calculates the time elapsed from a user-specified date to now, formatted with multiple units.
- Write a PHP script to display the detailed time difference between two events, using DateTime::diff() and formatting the output elegantly.
Go to:
PREV : Validate Given Dates.
NEXT : Convert Month Number to Month Name.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.