PHP for loop Exercises : Add all between two integers and display the sum
PHP for loop: Exercise-2 with Solution
Create a script using a for loop to add all the integers between 0 and 30 and display the sum.
Visual Presentation:
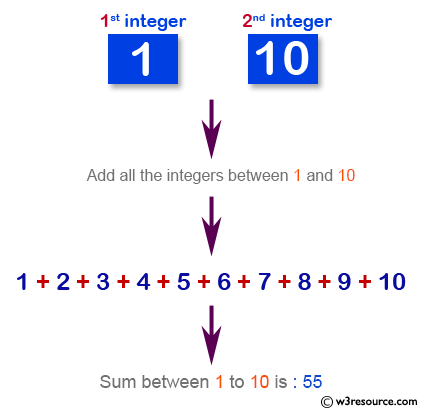
Sample Solution:
PHP Code:
<?php
// Initialize sum variable
$sum = 0;
// Loop through numbers from 1 to 30
for($x=1; $x<=30; $x++)
{
// Add current number to the sum
$sum += $x;
}
// Print the sum of numbers from 1 to 30
echo "The sum of the numbers 0 to 30 is $sum"."\n";
?>
Output:
The sum of the numbers 0 to 30 is 465
Explanation:
In the exercise above,
- The code starts with a PHP opening tag <?php.
- It initializes '$sum' to 0. This variable will store the sum of numbers from 1 to 30.
- The code enters a "for" loop that iterates through numbers from 1 to 30 (for($x=1; $x<=30; $x++)), incrementing the variable '$x' by 1 in each iteration.
- Within the loop, each value of '$x' is added to the '$sum' variable ($sum += $x;), effectively accumulating the sum of all the numbers from 1 to 30.
- After the loop, it prints out the calculated sum using echo, along with a message indicating what the sum represents (echo "The sum of the numbers 0 to 30 is $sum"."\n";).
- Finally, the PHP code ends with a closing tag ?>.
Flowchart :
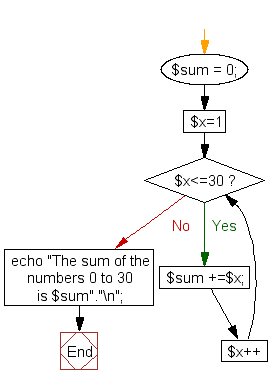
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Create a script that displays 1-2-3-4-5-6-7-8-9-10 on one line. There will be no hyphen(-) at starting and ending position.
Next: Create a script to construct the specific pattern, using nested for loop.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics