PHP Math Exercises: Get random float numbers
10. Generate Random Float Numbers
Write a PHP function to get random float numbers.
Pictorial Presentation:
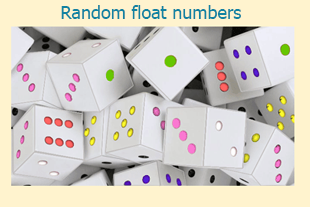
Sample Solution:
PHP Code:
<?php
function rand_float($st_num = 0, $end_num = 1, $mul = 1000000)
{
// Check if the start number is greater than the end number
if ($st_num > $end_num) {
return false; // Return false if start number is greater than end number
}
// Generate a random integer between the multiplied start and end numbers,
// then divide it by the multiplication factor to get a random float value
return mt_rand($st_num * $mul, $end_num * $mul) / $mul;
}
// Test the rand_float function with different arguments and print the results
echo rand_float() . "\n"; // Random float between 0 and 1
echo rand_float(0.6) . "\n"; // Random float between 0.6 and 1
echo rand_float(0.5, 0.6) . "\n"; // Random float between 0.5 and 0.6
echo rand_float(0, 20) . "\n"; // Random float between 0 and 20
echo rand_float(0, 3, 2) . "\n"; // Random float between 0 and 3
echo rand_float(0, 2, 20) . "\n"; // Random float between 0 and 2
?>
Output:
0.613314 0.886207 0.57955 17.570268 1.5 1.6
Explanation:
In the exercise above,
- function rand_float($st_num = 0, $end_num = 1, $mul = 1000000) {: This line defines a function named "rand_float()" with three parameters: '$st_num', '$end_num', and '$mul'. Default values are provided for these parameters.
- if ($st_num > $end_num) { return false; }: This line checks if the start number is greater than the end number. If it is, the function returns 'false'.
- return mt_rand($st_num $mul, $end_num $mul) / $mul;: This line generates a random integer between the multiplied start and end numbers (multiplied by the factor '$mul'), then divides it by the multiplication factor to get a random float value. This ensures the float value falls within the specified range.
- The subsequent "echo" statements test the "rand_float()" function with different arguments and print the results.
Flowchart :
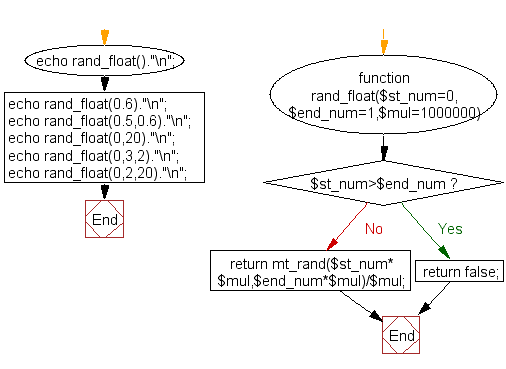
For more Practice: Solve these Related Problems:
- Write a PHP function to generate a random float between two given numbers without using the rand() function.
- Write a PHP script to simulate random float generation using microtime() for increased precision.
- Write a PHP program to generate an array of random floating-point numbers within a specified range and print the results.
- Write a PHP function to generate a random float with a given number of decimal places by manipulating string representations.
Go to:
PREV : Arabic to Roman Numerals Conversion.
NEXT : Human-Readable Captcha String.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.