PHP Math Exercises : Get the distance between two points on the earth
PHP math: Exercise-12 with Solution
Write a PHP function to get the distance between two points on the earth.
Pictorial Presentation:
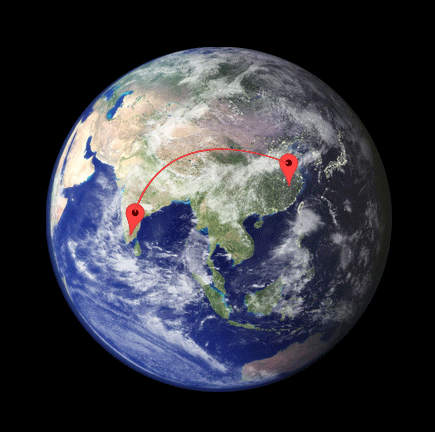
Sample Solution:
PHP Code:
<?php
// Define a function to calculate the distance between two points given their latitude and longitude
function lat_long_dist_of_two_points($latitudeFrom, $longitudeFrom, $latitudeTo, $longitudeTo){
// Define the value of pi
$pi = pi();
// Calculate the angular distance between the two points using Haversine formula
$x = sin($latitudeFrom * $pi/180) *
sin($latitudeTo * $pi/180) +
cos($latitudeFrom * $pi/180) *
cos($latitudeTo * $pi/180) *
cos(($longitudeTo * $pi/180) - ($longitudeFrom * $pi/180));
// Calculate the arc tangent
$x = atan((sqrt(1 - pow($x, 2))) / $x);
// Convert the angular distance to kilometers and then to miles
return abs((1.852 * 60.0 * (($x/$pi) * 180)) / 1.609344);
}
// Calculate the distance from New York to London and echo the result
echo lat_long_dist_of_two_points(40.7127, 74.0059, 51.5072, 0.1275).' mi'."\n";
?>
Output:
3458.8601748984 mi
Explanation:
In the exercise above,
- function lat_long_dist_of_two_points($latitudeFrom, $longitudeFrom, $latitudeTo, $longitudeTo){: This line defines a function named "lat_long_dist_of_two_points()" which takes four parameters: the latitude and longitude of the first point ('$latitudeFrom', '$longitudeFrom'), and the latitude and longitude of the second point ('$latitudeTo', '$longitudeTo').
- $pi = pi();: This line assigns the value of pi to the variable '$pi'.
- The subsequent lines determine the distance between the two points using the Haversine formula. This is a method used to calculate the distance between two points on a sphere.
- return abs((1.852 60.0 (($x/$pi) * 180)) / 1.609344);: This line calculates the distance between the two points in kilometers using the Haversine formula and converts it to miles.
- echo lat_long_dist_of_two_points(40.7127, 74.0059, 51.5072, 0.1275).' mi'."\n";: This line calls the "lat_long_dist_of_two_points()" function with the latitude and longitude coordinates of New York and London as arguments, calculates the distance between them, and echoes the result in the unit "mi" (miles).
Flowchart :
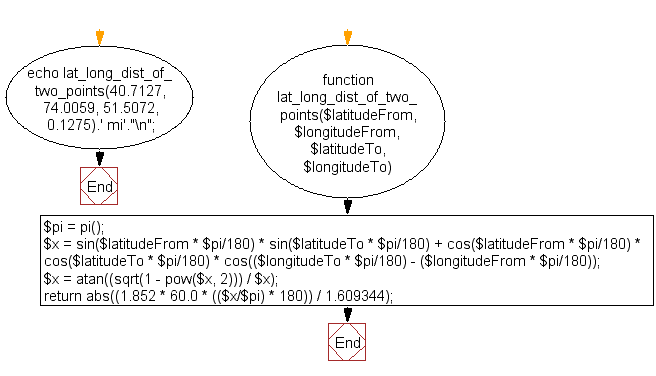
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a PHP function to create a human-readable random string for a captcha.
Next: PHP Classes Exercises Home.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics