PHP Regular Expression Exercise: Remove all characters from a string except a-z A-Z 0-9 or " "
7. Remove all characters from a string except a-z A-Z 0-9 or " "
Write a PHP script to remove all characters from a string except a-z A-Z 0-9 or " ".
Sample string: abcde$ddfd @abcd )der]
Visual Presentation:
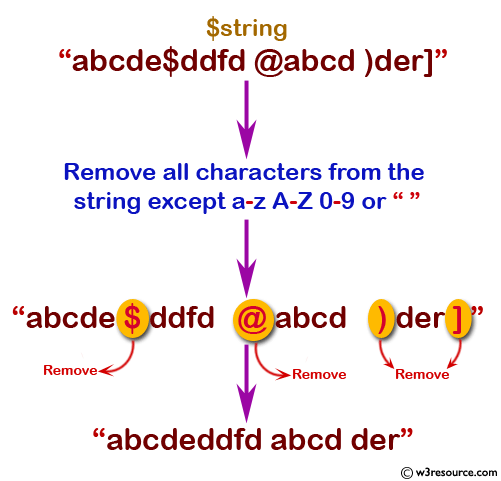
Sample Solution:
PHP Code:
<?php
// Define the input string
$string = 'abcde$ddfd @abcd )der]';
// Print the old string
echo 'Old string : '.$string.'';
// Remove all characters except letters, numbers, and spaces using regular expression
$newstr = preg_replace("/[^A-Za-z0-9 ]/", '', $string);
// Print the new string after removing unwanted characters
echo 'New string : '.$newstr."\n";
?>
Output:
Old string : abcde$ddfd @abcd )der] New string : abcdeddfd abcd der
Explanation:
The above PHP code takes a string as input, removes all characters except letters (both uppercase and lowercase), numbers, and spaces using a regular expression, and then prints both the original string and the modified string.
It achieves this by utilizing the "preg_replace()" function with a regular expression pattern that matches any character that is not a letter, number, or space, and replaces it with an empty string.
Finally, it prints both the original and modified strings.
Flowchart :
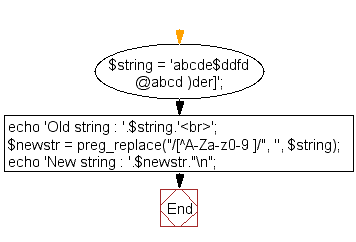
For more Practice: Solve these Related Problems:
- Write a PHP script to strip all special characters from a string, preserving only alphanumerics and spaces, and convert the result to lowercase.
- Write a PHP function to remove all punctuation from a string except spaces, then count the frequency of each remaining word.
- Write a PHP program to remove everything from a string except alphanumeric characters and single spaces, ensuring no multiple spaces remain.
- Write a PHP script to clean a string by removing characters not matching a specific whitelist: a-z, A-Z, 0-9, space, and selected Unicode characters.
Go to:
PREV : Extract text (within parenthesis) from a string.
NEXT : PHP Date Exercises Home.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.