Python Projects: Print the song 99 bottles of beer on the wall
Python Project-4 with Solution
Create a Python project that prints out every line of the song "99 bottles of beer on the wall."
Note: Try to use a built in function instead of manually type all the lines.
Sample Solution -1 :
Python Code:
# https://github.com/luongthomas/Python-Mini-Projects/blob/master/99%20Bottles/99bottles.py
# Purpose: A program printing out every line to the song "99 bottles of beer on the wall"
# Notes: Besides the phrase "take one down", can't manually type numbers/names into song
# When reached 1 bottle left, "bottles" becomes singular
def sing(n):
if (n == 1):
objects = 'bottle'
objectsMinusOne = 'bottles'
elif (n == 2):
objects = 'bottles'
objectsMinusOne = 'bottle'
else:
objects = 'bottles'
objectsMinusOne = 'bottles'
if (n > 0):
print(str(n) + " " + objects + " of beer on the wall, " + str(n) + " " + objects + " of beer.")
print("Take one down and pass it around, " + str(n-1) + " " + objectsMinusOne + " of beer on the wall.")
print(" ")
elif (n == 0):
print("No more bottles of beer on the wall, no more bottles of beer.")
print("Go to the store and buy some more, 99 bottles of beer on the wall.")
else:
print("Error: Wheres the booze?")
bottles = 99
while bottles >= 0:
sing(bottles)
bottles -= 1
Output:
99 bottles of beer on the wall, 99 bottles of beer. Take one down and pass it around, 98 bottles of beer on the wall. 98 bottles of beer on the wall, 98 bottles of beer. Take one down and pass it around, 97 bottles of beer on the wall. .......................................... 63 bottles of beer on the wall, 63 bottles of beer. Take one down and pass it around, 62 bottles of beer on the wall. 62 bottles of beer on the wall, 62 bottles of beer. Take one down and pass it around, 61 bottles of beer on the wall. .......................................... 2 bottles of beer on the wall, 2 bottles of beer. Take one down and pass it around, 1 bottle of beer on the wall. 1 bottle of beer on the wall, 1 bottle of beer. Take one down and pass it around, 0 bottles of beer on the wall. No more bottles of beer on the wall, no more bottles of beer. Go to the store and buy some more, 99 bottles of beer on the wall.
Flowchart:
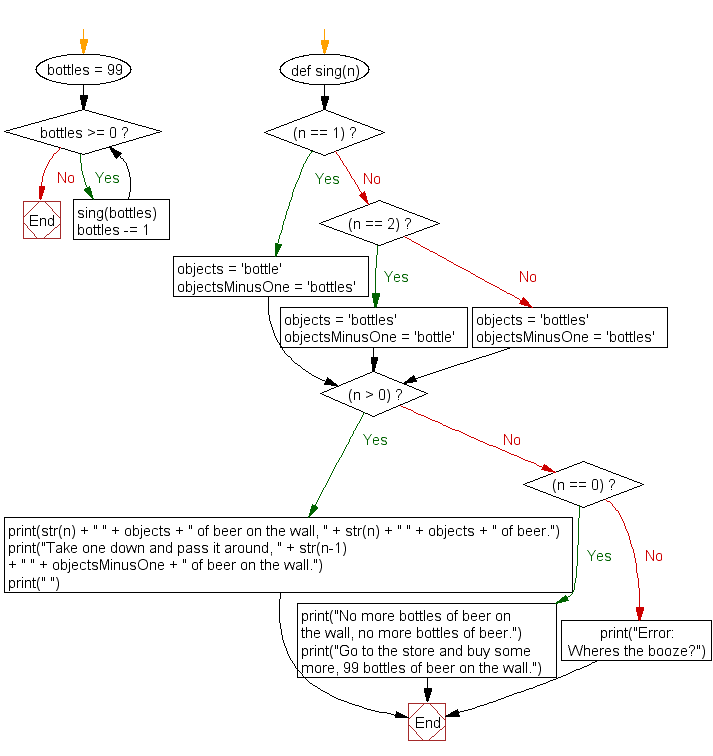
Sample Solution -2 :
99 bottles of beer...
Python Code:
import time
# https://github.com/marcgushwa/99bottles/blob/master/99.py
## 99 Bottles of beer on the wall
## 99 Bottles of beer
## Take one down, pass it around
## 98 bottles of beer on the wall
bottles = 99
while bottles > 0:
print(bottles,"bottles of beer on the wall")
print(bottles,"bottles of beer")
print("Take one down, pass it around")
bottles = bottles - 1
print(bottles,"bottles of beer on the wall")
time.sleep(1)
Sample Output:
99 bottles of beer on the wall 99 bottles of beer Take one down, pass it around 98 bottles of beer on the wall .............................. 62 bottles of beer on the wall 62 bottles of beer ............................. 2 bottles of beer on the wall 2 bottles of beer on the wall 2 bottles of beer Take one down, pass it around 1 bottles of beer on the wall 1 bottles of beer on the wall 1 bottles of beer Take one down, pass it around 0 bottles of beer on the wall
Flowchart:
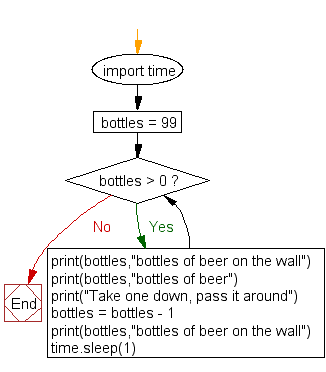
Sample Solution -3 :
99 bottles of beer...
Python Code:
# https://github.com/RianGallagher/Beginner-projects-solutions/blob/master/99bottles.py
for i in range(99, 0, -1):
if(i == 2):
print("{} bottles of beer on the wall, {} bottles of beer, take one down, pass it around, {} bottle of beer on the wall".format(i, i, i-1))
elif(i == 1):
print("{} bottle of beer on the wall, {} bottle of beer, take one down, pass it around, no bottles of beer on the wall".format(i, i))
else:
print("{} bottles of beer on the wall, {} bottles of beer, take one down, pass it around, {} bottles of beer on the wall".format(i, i, i-1))
Sample Output:
99 bottles of beer on the wall, 99 bottles of beer, take one down, pass it around, 98 bottles of beer on the wall 98 bottles of beer on the wall, 98 bottles of beer, take one down, pass it around, 97 bottles of beer on the wall ........................ 61 bottles of beer on the wall, 61 bottles of beer, take one down, pass it around, 60 bottles of beer on the wall ........................ 2 bottles of beer on the wall, 2 bottles of beer, take one down, pass it around, 1 bottle of beer on the wall 1 bottle of beer on the wall, 1 bottle of beer, take one down, pass it around, no bottles of beer on the wall
Flowchart:
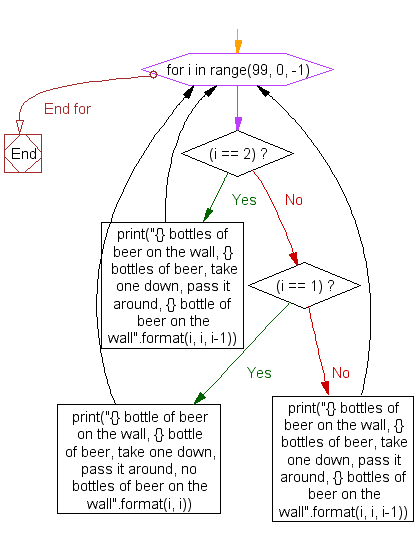
Sample Solution -4 :
99 bottles of beer...
Python Code:
# https://github.com/Everstalk/BP/blob/master/99%20Bottles.py
def counter():
for i in range(99,-1,-1):
if i == 0:
print ("No more bottles of beer on the wall, no more bottles of beer.")
print ("Go to the store and buy some more,99 bottles of beer on the wall")
elif i == 1:
print ("1 bottle of beer on the wall, 1 bottle of beer.")
print ("Take one down and pass it around, no more bottles of beer to go")
elif i == 2:
print (i," bottles of beer on the wall, ",i," bottles of beer.")
print ("Take one down and pass it around, ",i-1," bottle of beer on the wall.")
else :
print (i," bottles of beer on the wall, ",i," bottles of beer.")
print ("Take one down and pass it around, ",i-1," bottles of beer on the wall.")
counter()
Sample Output:
99 bottles of beer on the wall, 99 bottles of beer. Take one down and pass it around, 98 bottles of beer on the wall. 98 bottles of beer on the wall, 98 bottles of beer. ..................................... 61 bottles of beer on the wall, 61 bottles of beer. Take one down and pass it around, 60 bottles of beer on the wall. 60 bottles of beer on the wall, 60 bottles of beer. ..................................... 1 bottle of beer on the wall, 1 bottle of beer. Take one down and pass it around, no more bottles of beer to go No more bottles of beer on the wall, no more bottles of beer. Go to the store and buy some more,99 bottles of beer on the wall
Flowchart:
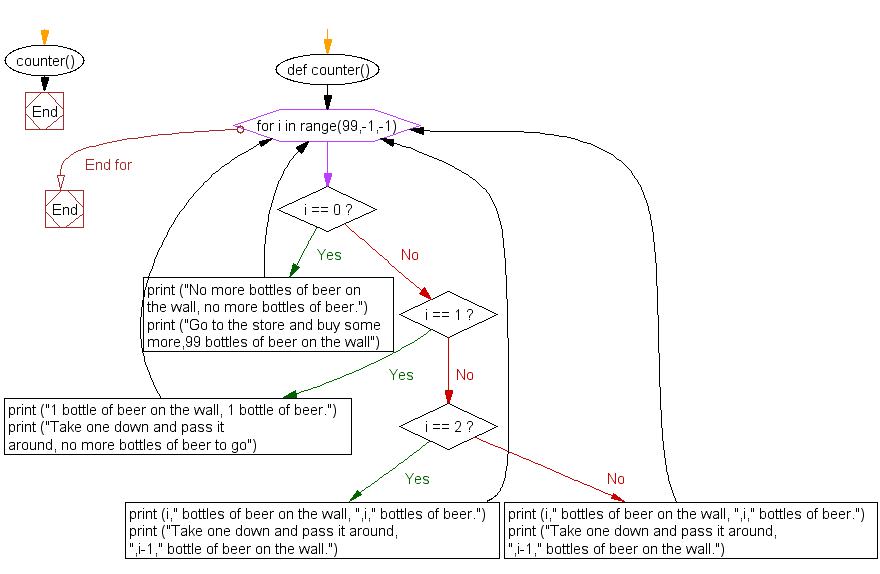
Improve this sample solutions and post your code through Disqus
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/projects/python/python-projects-4.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics