Python Projects: Magic 8 Ball for fortune-telling or seeking advice
Python Project-5 with Solution
Create a Python project of a Magic 8 Ball which is a toy used for fortune-telling or seeking advice.
Note :
- Allow the user to input their question.
- Show an in progress message.
- Create 10/20 responses, and show a random response.
- Allow the user to ask another question/advice or quit the game.
Sample Solution -1 :
Python Code:
#Make a Magic 8 ball
#https://github.com/viljow/magic8/blob/master/main.py
import random
answers = ['It is certain', 'It is decidedly so', 'Without a doubt', 'Yes – definitely', 'You may rely on it', 'As I see it, yes', 'Most likely', 'Outlook good', 'Yes Signs point to yes', 'Reply hazy', 'try again', 'Ask again later', 'Better not tell you now', 'Cannot predict now', 'Concentrate and ask again', 'Dont count on it', 'My reply is no', 'My sources say no', 'Outlook not so good', 'Very doubtful']
print(' __ __ _____ _____ _____ ___ ')
print(' | \/ | /\ / ____|_ _/ ____| / _ \ ')
print(' | \ / | / \ | | __ | || | | (_) |')
print(' | |\/| | / /\ \| | |_ | | || | > _ < ')
print(' | | | |/ ____ \ |__| |_| || |____ | (_) |')
print(' |_| |_/_/ \_\_____|_____\_____| \___/ ')
print('')
print('')
print('')
print('Hello World, I am the Magic 8 Ball, What is your name?')
name = input()
print('hello ' + name)
def Magic8Ball():
print('Ask me a question.')
input()
print (answers[random.randint(0, len(answers)-1)] )
print('I hope that helped!')
Replay()
def Replay():
print ('Do you have another question? [Y/N] ')
reply = input()
if reply == 'Y':
Magic8Ball()
elif reply == 'N':
exit()
else:
print('I apologies, I did not catch that. Please repeat.')
Replay()
Magic8Ball()
Output:
__ __ _____ _____ _____ ___ | \/ | /\ / ____|_ _/ ____| / _ \ | \ / | / \ | | __ | || | | (_) | | |\/| | / /\ \| | |_ | | || | > _ < | | | |/ ____ \ |__| |_| || |____ | (_) | |_| |_/_/ \_\_____|_____\_____| \___/ Hello World, I am the Magic 8 Ball, What is your name? Sara hello Sara Ask me a question. Tell my fortune It is certain I hope that helped! Do you have another question? [Y/N] Y Ask me a question. My favorite color My reply is no I hope that helped! Do you have another question? [Y/N] N
Flowchart:
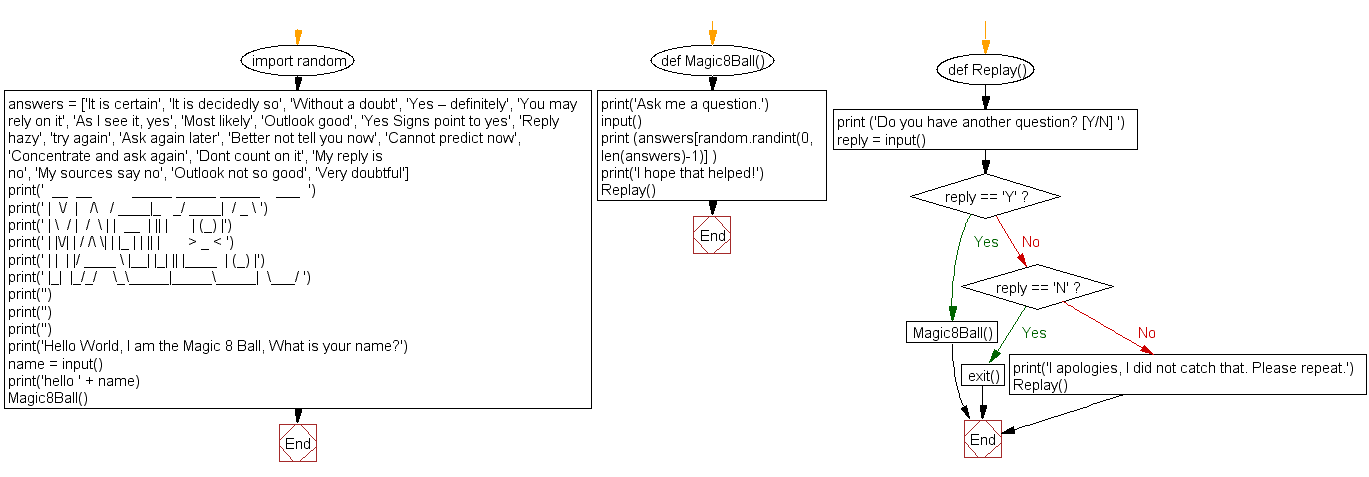
Sample Solution -2 :
Python Code:
#https://github.com/soupyck/Magic8Ball/blob/master/magic8ball.py
import random
import time
eight_ball = [ "It is certain", "It is decidedly so", "Without a doubt", "Yes, definitely",
"You may rely on it", "As I see it, yes", "Most Likely", "Outlook Good",
"Yes", "Signs point to yes", "Reply hazy, try again", "Ask again later",
"Better not tell you now", "Cannot predict now", "Concentrate and ask again",
"Don't count on it", "My reply is no", "My sources say no", "Outlook not so good", "Very Doubtful"]
def question():
question = input("You may ask your yes or no question of the Magic 8 Ball!\n")
print("Thinking...")
time.sleep(random.randrange(0,5))
print(random.choice(eight_ball))
while True:
question()
repeat = input("Would you like to ask another question? (Y or N)")
if not (repeat == "y" or repeat == "Y"):
print("Come back if you have more questions!")
break
Sample Output:
You may ask your yes or no question of the Magic 8 Ball! Tell me my fortune Thinking... You may rely on it Would you like to ask another question? (Y or N)Y You may ask your yes or no question of the Magic 8 Ball! yes Thinking... Yes, definitely Would you like to ask another question? (Y or N)n Come back if you have more questions!
Flowchart:
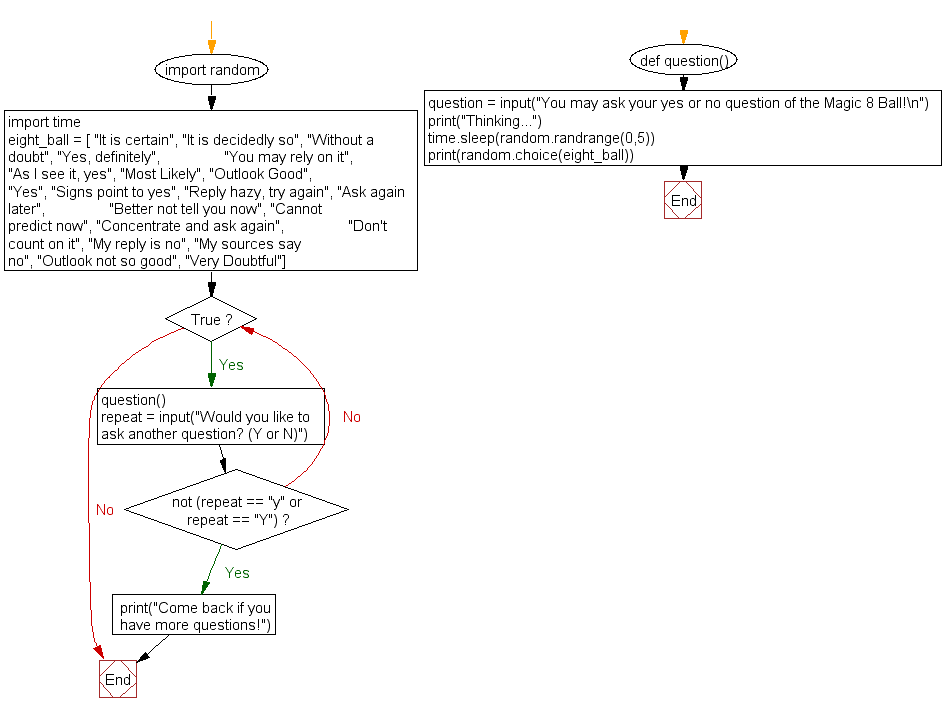
Sample Solution -3 :
99 bottles of beer...
Python Code:
https://github.com/pixelnull/8ball/blob/master/8ball.py
import random
import time
# Set count to how many times user wants to do the magic 8ball
count_str = input("Hello user. How many questions would you like to ask the 8-Ball? ")
# Handling if count_str is not a number
while True:
if count_str.isdigit():
count = int(count_str)
break
else:
count_str = input("You cheeky devil, just enter a simple number this time: ")
# Outlier input handling
if count <= 0:
print("Well I didn't want to play with you either!")
time.sleep(5)
exit()
elif count > 10:
print("\nYou have to be kidding me, you want to do that many questions?\nWe'll do 1 and go from there.\n", sep='')
count = 1
# Sequence for random.choice
answers = ["It is certain.", "It is decidedly so.", "Without a doubt", "Yes definitely.", "You may rely on it.",
"As I see it, yes.", "Most likely.", "Outlook good.", "Yes.", "Signs point to yes.", "Reply hazy try again.",
"Ask again later.", "Better not tell you now.", "Cannot predict now.", "Concentrate and ask again.",
"Don\'t count on it.", "My reply is no.", "My sources say no.", "Outlook not so good.", "Very doubtful."]
# Main loop and graceful exit
while count > 0:
blah = input("Type your question: ")
dice = random.choice(answers)
print("\n", dice, "\n", sep='')
time.sleep(2)
count = count - 1
else:
print("\nI hope you liked the answer(s). THE GREAT 8-BALL HAS SPOKEN!")
time.sleep(5)
exit()
Sample Output:
Hello user. How many questions would you like to ask the 8-Ball? 1 Type your question: Tell me my fortune Signs point to yes. I hope you liked the answer(s). THE GREAT 8-BALL HAS SPOKEN!
Flowchart:
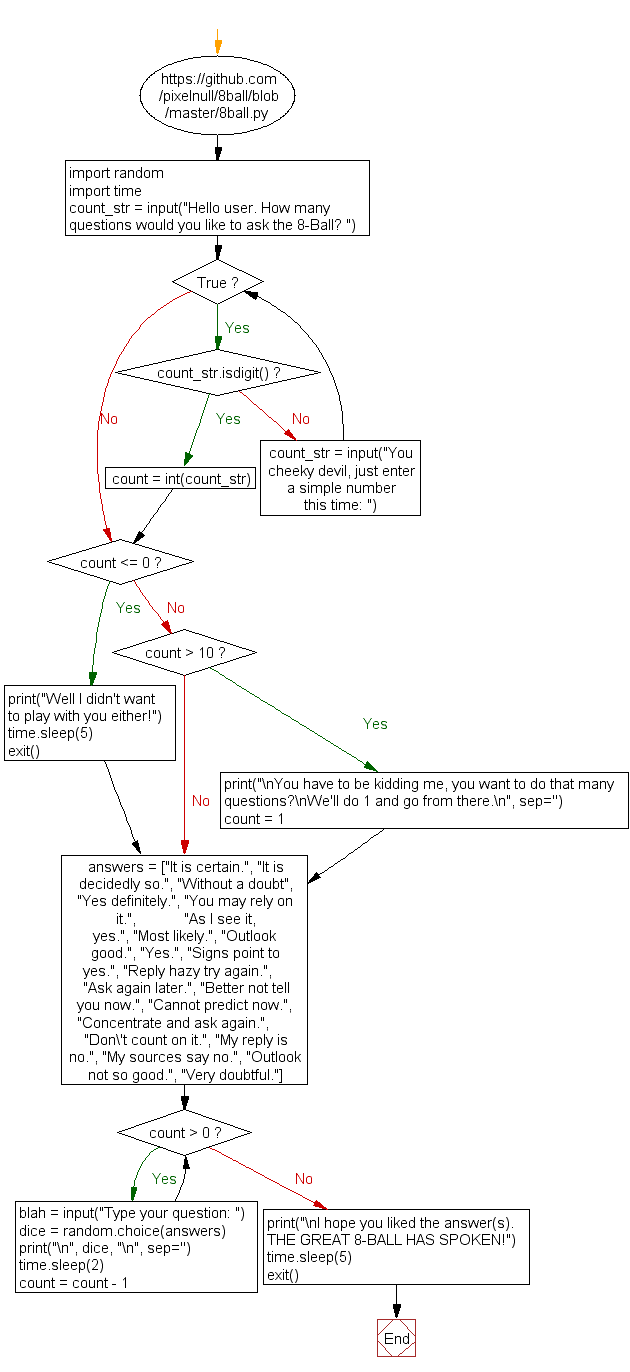
Sample Solution -4 :
Python Code:
#https://github.com/DeronKHolmes/magic-8-ball-game/blob/master/eightball.py
import random
import time, sys
play_again = 'yes'
while play_again == 'yes':
str(input("Welcome to the Magic 8-Ball. Enter your question: ")).lower()
#Delay output for 1 second each
print("Thinking...")
time.sleep(1)
print("3...")
time.sleep(1)
print("2...")
time.sleep(1)
print("1...")
time.sleep(1)
print()
#Generate a random integer/response
response = random.randint(0,20)
if response == 1:
print("Not just no, hell no!")
elif response == 2:
print("Sure thing.")
elif response == 3:
print("Don't count on it.")
elif response == 4:
print("Maybe not.")
elif response == 5:
print("Count on it.")
elif response == 6:
print("The Universe says maybe.")
elif response == 7:
print("I don't see why not.")
elif response == 8:
print("The future looks good for you.")
elif response == 9:
print("That's for sure.")
elif response == 10:
print("Maybe.")
elif response == 11:
print("There's a chance.")
elif response == 12:
print("Certainly!")
elif response == 13:
print("Keep doing what you're doing and it'll happen.")
elif response == 14:
print("Not over my dead 8 Ball.")
elif response == 15:
print("No.")
elif response == 16:
print("Yes.")
elif response == 17:
print("All depends on if you've been good for Santa this year.")
elif response == 18:
print("Not in this lifetime.")
elif response == 19:
print("Someday, but not today.")
elif response == 20:
print("Right after you hit the lottery.")
else:
print("Not a valid question!")
play_again = str(input("Would you like to ask another question? yes/no ")).lower()
if play_again == 'no':
print("Goodbye! Thanks for playing!")
sys.exit()
Sample Output:
Welcome to the Magic 8-Ball. Enter your question: Tell me my fortune Thinking... 3... 2... 1... Maybe not. Would you like to ask another question? yes/no yes Welcome to the Magic 8-Ball. Enter your question: My favorite color Thinking... 3... 2... 1... No. Would you like to ask another question? yes/no no Goodbye! Thanks for playing!
Flowchart:
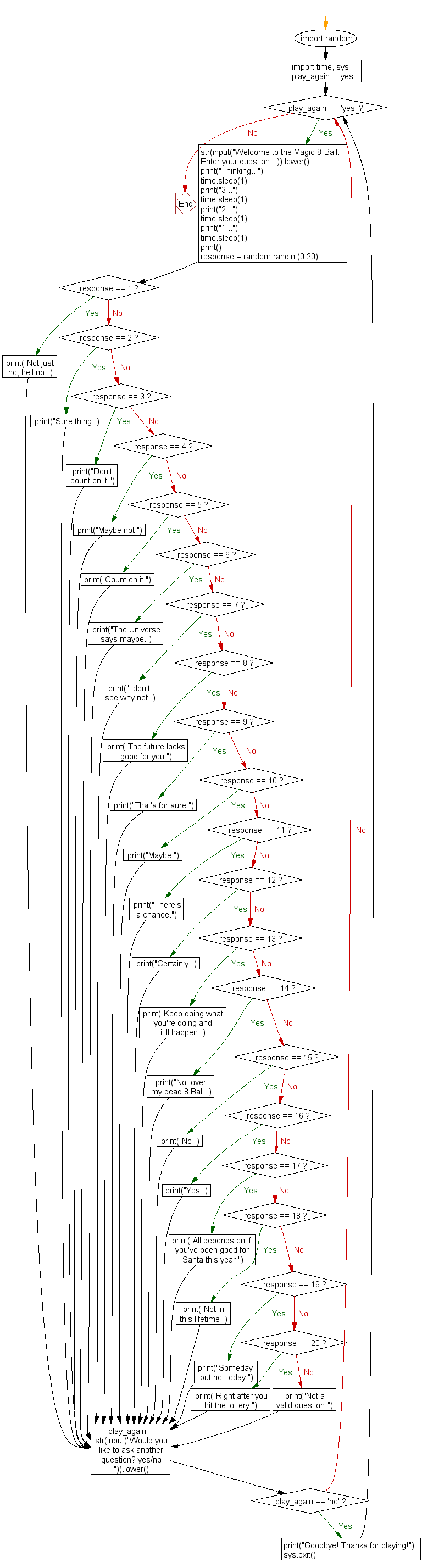
Contribute your code and comments through Disqus.