Python: Find the longest common ending between two given strings
Longest Common Ending
Write a Python program to find the longest common ending between two given strings.
Sample Solution-1:
Python Code:
# Define a function 'test' that takes two strings as input.
def test(str1, str2):
# Iterate through the indices of the second string.
for i in range(len(str2)):
# Check if a substring of the second string starting from index 'i' is present at the end of the first string,
# and if the last characters of both strings match.
while str2[i:] in str1 and str2[-1] == str1[-1]:
# If the conditions are met, return the common ending substring.
return str2[i:]
# If no common ending is found, return an empty string.
return ""
# Test case 1
str1_1 = "running"
str2_1 = "ruminating"
print("Original strings: " + str1_1 + " " + str2_1)
print("Common ending between said two strings: " + test(str1_1, str2_1))
# Test case 2
str1_2 = "thisisatest"
str2_2 = "testing123testing"
print("\nOriginal strings: " + str1_2 + " " + str2_2)
print("Common ending between said two strings: " + test(str1_2, str2_2))
Sample Output:
Original strings: running ruminating Common ending between said two strings: ing Original strings: thisisatest testing123testing Common ending between said two strings:
Explanation:
Here is a breakdown of the above Python code:
- test Function:
- The "test()" function takes two strings as input.
- It iterates through the indices of the second string (str2).
- For each index 'i', it checks if a substring of 'str2' starting from index i is present at the end of 'str1', and if the last characters of both strings match.
- If these conditions are met, it returns the common ending substring.
- If no common ending is found, it returns an empty string.
Flowchart:
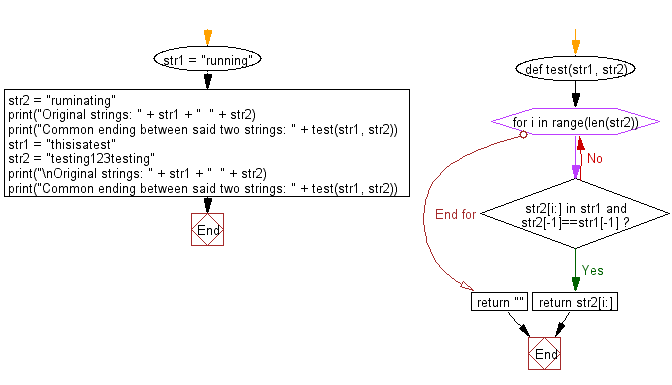
Sample Solution-2:
Python Code:
# Define a function 'test' that takes two strings as input.
def test(str1, str2):
# Continue removing the first character of str2 until it becomes a suffix of str1.
while not str1.endswith(str2):
str2 = str2[1:]
# Return the common ending between str1 and str2.
return str2
# Test case 1
str1_1 = "running"
str2_1 = "ruminating"
print("Original strings: " + str1_1 + " " + str2_1)
print("Common ending between said two strings: " + test(str1_1, str2_1))
# Test case 2
str1_2 = "thisisatest"
str2_2 = "testing123testing"
print("\nOriginal strings: " + str1_2 + " " + str2_2)
print("Common ending between said two strings: " + test(str1_2, str2_2))
Sample Output:
Original strings: running ruminating Common ending between said two strings: ing Original strings: thisisatest testing123testing Common ending between said two strings:
Explanation:
Here is a breakdown of the above Python code:
- test Function:
- The "test()" function takes two strings as input.
- It uses a while loop to repeatedly remove the first character of 'str2' until 'str2' becomes a suffix of 'str1'.
- When the loop condition (not str1.endswith(str2)) is 'False', the function returns the common ending between 'str1' and 'str2'.
Flowchart:
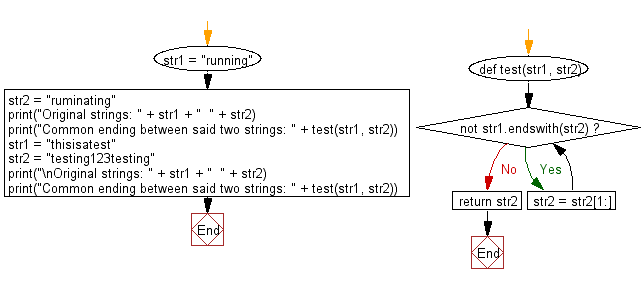
For more Practice: Solve these Related Problems:
- Write a Python program to find the longest common suffix between two strings by comparing characters from the end.
- Write a Python program to determine the common ending substring of two input strings.
- Write a Python program to reverse both strings and compute the longest common prefix of the reversed strings.
- Write a Python program to extract and return the longest common ending shared by two given strings.
Go to:
Previous: Write a Python program to reverse all the words which have odd length.
Next: Write a Python program to reverse the binary representation of an given integer and convert the reversed binary number into an integer.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.