Python: Convert all items in a given list to float values
Python Basic - 1: Exercise-140 with Solution
Write a Python program to convert all items in a given list to float values.
Sample Solution-1:
Python Code:
# Define a list 'nums' containing string representations of decimal numbers.
nums = ['0.49', '0.54', '0.54', '0.54', '0.54', '0.54', '0.55', '0.54', '0.54', '0.54',
'0.55', '0.55', '0.55', '0.54', '0.55', '0.55', '0.54', '0.55', '0.55', '0.54']
# Display the original list.
print("Original list:")
print(nums)
# Display a message indicating the next section.
print("\nList of Floats:")
# Create an empty list 'nums_of_floats' to store float representations of the decimal numbers.
nums_of_floats = []
# Iterate through each item in the original list.
for item in nums:
# Convert each string representation to a float and append it to 'nums_of_floats'.
nums_of_floats.append(float(item))
# Display the list of floats.
print(nums_of_floats)
Sample Output:
Original list: ['0.49', '0.54', '0.54', '0.54', '0.54', '0.54', '0.55', '0.54', '0.54', '0.54', '0.55', '0.55', '0.55', '0.54', '0.55', '0.55', '0.54', '0.55', '0.55', '0.54'] List of Floats: [0.49, 0.54, 0.54, 0.54, 0.54, 0.54, 0.55, 0.54, 0.54, 0.54, 0.55, 0.55, 0.55, 0.54, 0.55, 0.55, 0.54, 0.55, 0.55, 0.54]
Explanation:
Here is a breakdown of the above Python code:
- Original List:
- The variable 'nums' is defined as a list containing string representations of decimal numbers.
- List of Floats:
- An empty list, 'nums_of_floats', is created to store float representations of the decimal numbers.
- A for loop iterates through each item in the original list (nums).
- Each string item is converted to a float using the "float()" function, and the result is appended to 'nums_of_floats'.
- The final list of float numbers (nums_of_floats) is displayed.
Flowchart:
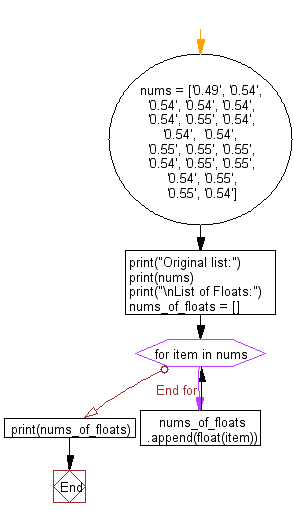
Sample Solution-2:
Python Code:
# Define a list 'nums' containing string representations of decimal numbers.
nums = ['0.49', '0.54', '0.54', '0.54', '0.54', '0.54', '0.55', '0.54', '0.54', '0.54',
'0.55', '0.55', '0.55', '0.54', '0.55', '0.55', '0.54', '0.55', '0.55', '0.54']
# Display the original list.
print("Original list:")
print(nums)
# Display a message indicating the next section.
print("\nList of Floats:")
# Use a list comprehension to create a list 'nums_of_floats' containing float representations of the decimal numbers.
nums_of_floats = [float(item) for item in nums]
# Display the list of floats.
print(nums_of_floats)
Sample Output:
Original list: ['0.49', '0.54', '0.54', '0.54', '0.54', '0.54', '0.55', '0.54', '0.54', '0.54', '0.55', '0.55', '0.55', '0.54', '0.55', '0.55', '0.54', '0.55', '0.55', '0.54'] List of Floats: [0.49, 0.54, 0.54, 0.54, 0.54, 0.54, 0.55, 0.54, 0.54, 0.54, 0.55, 0.55, 0.55, 0.54, 0.55, 0.55, 0.54, 0.55, 0.55, 0.54]
Explanation:
Here is a breakdown of the above Python code:
- Original List:
- The variable 'nums' is defined as a list containing string representations of decimal numbers.
- List of Floats:
- A list comprehension is used to create a new list, 'nums_of_floats', containing float representations of the decimal numbers.
- The final list of float numbers (nums_of_floats) is displayed.
Flowchart:
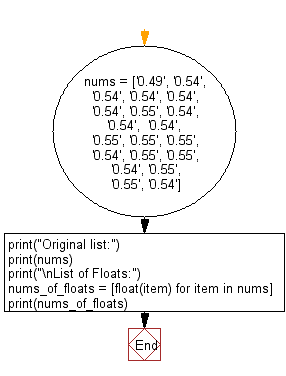
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to find the closest palindrome number of a given integer. If there are two palindrome numbers in absolute distance return the smaller number.
Next: Write a Python program to get the domain name using PTR DNS records from a given IP address.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics